One of the common tasks in programming is to manipulate dates and times. Sometimes, we need to add days to a current/given date to get a specific date. For example, we may want to calculate the expiration date of a subscription, the due date of a task, or the delivery date of an order. Don’t know how to do that? No worries! This Java guide illustrates several methods to add days to any given date or current date using easily understandable examples.
How to Add Days to a Date in Java
To add days to a given/current date, you can use the built-in Java classes like Calendar, LocalDate, and Instant. Also, you can use the Apache Commons Library to add days to date in Java.
How to Add Days to a Date Through Java Calendar Class
Calendar is an abstract class in Java that offers several methods to work with the date and time data. add() is a useful method of this class that helps us add days to the current date, specific date, or any user-specified date. It accepts a “calendar field” and “number of days” to be added as parameters
cal.add(Calendar.DATE, no_of_days);
It adds the days to the date according to calendar rules, such as
- The month must be <= 12
- The year must be in 4 digits
- The days should be <= 28, 29, 30, or 31 (depending on the month).
Let’s understand this method via an example.
Example 1: Adding 5 Days to a Specific Date
In the following code snippet, first, we import the classes from the text and util packages:
package javaexamples;
//import required classes like SimpleDateFormat, ParseException, and Calendar
import java.text.*;
import java.util.Calendar;
class AddDays{
public static void main(String args[]){
//Declare and Print the Actual Date
String inputDate = "01/12/2020";
System.out.println("The Actual Date is: " + inputDate);
//Specify a Valid Date Format to Match the Given Date
SimpleDateFormat dateFormat = new SimpleDateFormat("MM/dd/yyyy");
//Set the calendar object according to the given Date
Calendar calendar = Calendar.getInstance();
try{
calendar.setTime(dateFormat.parse(inputDate));
}catch(ParseException excep){
excep.printStackTrace();
}
//Add Days to Date Using add() Method
calendar.add(Calendar.DAY_OF_MONTH, 5);
String modifiedDate = dateFormat.format(calendar.getTime());
//Print the Modified Date
System.out.println("The Modified Date is: " + modifiedDate);
}
}
In the main() method:
- We declare a string and initialize it with a date value in “MM/dd/yyyy” format.
- After this, we use the SimpleDateFormat() constructor and pass it a valid date format “MM/dd/yyyy” to match the given date.
- We set the time of the Calendar object according to the given Date.
- Within the try block, we parse the given date string into a date object (according to the specified format).
- In the catch block, we use the printStackTrace() to deal with the possible parsing exceptions.
- Finally, we use the add() method of the Calendar class to add days to a date and print it on the console:

Example 2: Adding 5 Days to the Current Date
In this example, all the code remains the same as in example 1. The only difference is that we add five days to the current date instead of a specific date:
package javaexamples;
//import required classes like SimpleDateFormat, ParseException, and Calendar
import java.text.*;
import java.util.Calendar;
class AddDays{
public static void main(String args[]){
//Specify a Valid Date Format to Match the Given Date
SimpleDateFormat dateFormat = new SimpleDateFormat("MM/dd/yyyy");
//Get the Current Date
Calendar calendar = Calendar.getInstance();
System.out.println("The Current Date: " + dateFormat.format(calendar.getTime()));
//Add Days to Date Using add() Method
calendar.add(Calendar.DAY_OF_MONTH, 5);
String modifiedDate = dateFormat.format(calendar.getTime());
//Print the Modified Date
System.out.println("The Modified Date is: " + modifiedDate);
}
}
To verify the working of the stated method, the current and updated dates are printed on the console:

How to Add Days to a Date Using LocalDate Class
Another convenient way of adding days to date is the use of the LocalDate class. This is an immutable Java class that expresses the date in “YYYY-MM-DD” format. This class offers a very useful date manipulation method named “plusDays()”. This method accepts the number of days as a parameter and adds them to the given or current date.
date.plusDays(no_of_days);
Note: the LocalDateTime class also offers the plusDays() method that adds the specified number of days into a date.
Example 1: Adding Specific Days to the Given Date
In this example, we import the LocalDate class and use its “of()” method to get a specific date:
package javaexamples;
//import the LocalDate classes
import java.time.LocalDate;
public class AddDays {
public static void main(String args[]) {
//Get and Print a Specific Date
LocalDate inputDate = LocalDate.of(2021, 06, 23);
System.out.println("Given Date is: " + inputDate);
//print the modified Date
System.out.println("Date After 12 Days is: " + inputDate.plusDays(12));
}
}
We use the plusDays() method with the days to be added as its argument. As a result, we get the output as follows:

Example 2: Adding Specific Days to the Current Date
In this example, we use the now() method of the LocalDate class to get the current date:
package javaexamples;
//import the LocalDate class
import java.time.LocalDate;
public class AddDays {
public static void main(String args[]) {
//Get and Print the Current Day
LocalDate currentDat = LocalDate.now();
System.out.println("Today is: " + currentDat);
//Get and Print Tomorrow's Date
LocalDate afterOneDay = currentDat.plusDays(1);
System.out.println("Day after Today: " + afterOneDay);
//Get and Print Date After Ten Days
LocalDate afterTenDays = currentDat.plusDays(10);
System.out.println("10 Days After Today: " + afterTenDays);
}
}
We use the plusDays() method to add the desired number of days to the current date:

How to Add Days to a Date Using Instant Class
The “Instant” class of the “java.time” package offers a “toInstant()” method that retrieves the current instant(date and time). We can execute this method with the “plus()” method to add the selected days to the current date. To execute this method, implement the following syntax:
currentInstant.plus(numberOfDays,ChronoUnit.DAYS);
Example: Adding Specific Days to the Current Date
In this example, first, we import the necessary classes like Instant, Date, and ChronoUnit enum:
package javaexamples;
//import the required classes
import java.time.Instant;
import java.util.Date;
import java.time.temporal.ChronoUnit;
class AddDays {
public static void main(String args[]) {
// Get and Print the Current Day
Date dat = new Date();
Instant currentInstant = dat.toInstant();
System.out.println("Current Date: " + currentInstant);
// Get and Print Date After 5 Days
Instant after5Days = currentInstant.plus(5, ChronoUnit.DAYS);
System.out.println("5 Days After Today: " + after5Days);
}
}
In the main() method, we get and print the current date using the toInstant() method. Up next, we use the plus() method to add the specified days to the current date and print the date after 5 days on the console:

How to Add Days to a Date Using Apache Commons Library
Apache Commons is an open-source library used to perform different basic to advanced tasks. You can use the DateUtils class of the Apache Commons to add specific days to a date. To use this class, you need to add the corresponding dependency into the “pom” file of your Maven project:
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.14.0</version>
</dependency>
</dependencies>

Once the dependency is successfully added to your project, import the desired classes like DateUtils and Date:
package mavenExample;
//importing required classes like DateUtils and Date
import org.apache.commons.lang3.time.DateUtils;
import java.util.Date;
public class AddDays {
public static void main(String[] args) {
//get and print current date
Date currentDat = new Date();
System.out.println("The Current Date: " + currentDat);
//get and print modified date
Date newDate = DateUtils.addDays(currentDat, 7);
System.out.println("Date After Adding 7 Days: " + newDate);
}
}
Now, use the “Date()” constructor to get the current date and print it on the console. After this, use the addDays() method of the DateUtils class to add the desired number of days to the current date:

How to Subtract Days From a Date
Java allows us to subtract a specific number of days from a date using different classes, such as Calendar, LocalDate, etc. In the following example, we use the minusDays() method of the LocalDate class to subtract days from a date:
package javaexamples;
//importing the LocalDate class
import java.time.LocalDate;
public class SubtractDay{
public static void main(String args[]) {
//Get and Print the Current Day
LocalDate currentDat = LocalDate.now();
System.out.println("Today is: " + currentDat);
//Get and Print Yesterday's Date
LocalDate beforeOneDay = currentDat.minusDays(1);
System.out.println("Day Before Today: " + beforeOneDay);
//Get and Print Date Before Ten Days
LocalDate beforeTenDays = currentDat.minusDays(10);
System.out.println("10 Days Before Today: " + beforeTenDays);
//Get and Print a Specific Date
LocalDate inputDate = LocalDate.of(2021, 06, 23);
System.out.println("Given Date is: " + inputDate);
//Modified Date
System.out.println("12 Days Before the Specified Date: " + inputDate.minusDays(12));
}
}
In this example, we implement the minusDays() method on different date values, including current date, and a specific date. The output shows that this method successfully subtracted the desired number of days from the given date values:
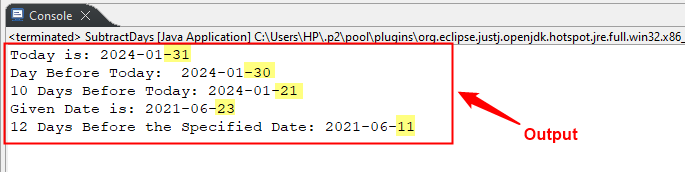
That’s all about adding days to a date in Java.
Conclusion
To add days to a date in Java, use the built-in classes like Calendar, LocalDate, Instant, or the DateUtils class of the Apache Commons Library. The “Calendar.add()” and “LocalDate.plusDays()” are the most popularly used methods as they can add days to the current date, specific date, or any user-specified date. Similarly, the “plus()” method of the “Instant” class is used with the “toInstant()” method to add the desired number of days to the current date. This blog post has demonstrated all these methods with suitable examples to add days to a date in Java.