In Java, a method is a group/block of statements combined to perform a specific task. It can be built-in or user-defined. No matter what type of a method is, it gets executed only when someone calls it. For instance, if we call a built-in “concat()” method, it combines the given strings. Similarly, if we create a user-defined method to find the square of two numbers, it will do this when we invoke it with its name.
Now without wasting any time, let’s dive in to learn the method creation and calling in Java using appropriate examples.
How to Create or Declare a Method in Java
The method creation or declaration consists of different components, such as method name, parameter list, access modifier, etc. Some components are compulsory while others are optional. Consider the following syntax to get a profound understanding of method creation:
accessModifier returnType methodName (parameterList)
{
//methodBody
}
“accessModifier”: It is an optional component of method creation that describes the access range of the method (from where you can access a method). A method can have one of the following modifiers:
Access Modifier | Description |
---|---|
Default | Accessible within the same class and package in which it is defined (not accessible from other packages). It is defined without explicitly specifying any modifier. |
Public | Accessible throughout your application, i.e., in all classes and subclasses (it is accessible from other packages as well). |
Protected | Accessible/Available in a class in which it is defined and in its child/subclasses. Also, you can access it in different classes of the same package. |
Private | It is accessible/callable only in the same class (in which it’s defined). |
“Static Keyword”: It is an optional component, if specified, the method can be accessed from the class without creating/using the class instance. If not specified, then the method will be accessed using the class object.
“returnType”: It is a mandatory component of method declaration, which must be one of the following: “any valid data type” or “void”. Where the void keyword represents that this method does not return anything.
“methodName”: It is a mandatory component of the method creation and it must be a valid name. Once a method is created, this name will be used to access or call it.
“parameterList”: It is an optional component and can be skipped. It represents how many parameters the declared method will accept. Parameters are specified with a valid data type and using comma-separated syntax.
“{methodBody}”: The method body is enclosed in curly braces. This is where you define your logic or write code to perform any specific operation. Whenever you call a method, the body gets executed and returns the results accordingly.
The following figure illustrates all the components of method declaration using a simple example:

How to Call a User-Defined Method in Java
Once a method is successfully created, you can call it from the main() method or from any other method. If a method is declared “static”, you can call/invoke it directly. However, if it’s not static, you must call it with the class instance.
Example 1: How to Create and Call a Static Method?
Let’s create a static method that accepts a parameter and returns the square of given numbers:
package javaBeat;
public class Examples {
public static int findSquare(int num1) {
int result;
result = num1 * num1;
return result;
}
public static void main(String[] args) {
System.out.println("The Square: " + findSquare(12));
}
}
We create a static method and name it findSquare(). It accepts an integer parameter num1. Within the method, we declare a new integer type “result” variable to store the square of the given number. We use the “*” symbol to compute the square of a specified integer. Since the method was declared static, so we invoke it in the main() method without the class instance. We invoke the findSquare() method within the println() method to print the desired outcome on the console:

Example 2: How to Create and Call a Non-Static Method
Now let’s create a non-static “subtractNumbers()” method that accepts two parameters and returns the difference between the given numbers:
package javaBeat;
public class Examples {
public int subtractNumbers(int num1, int num2) {
int result;
result = num2 - num1;
return result;
}
public static void main(String[] args) {
Examples obj = new Examples();
System.out.println("num2 - num1: " + obj.subtractNumbers(12, 100));
}
}
In the main() method, first, we create an object of the Examples class using the new operator. In the next line, we use that object with the dot syntax to invoke the subtractNumbers() method with a couple of arguments:
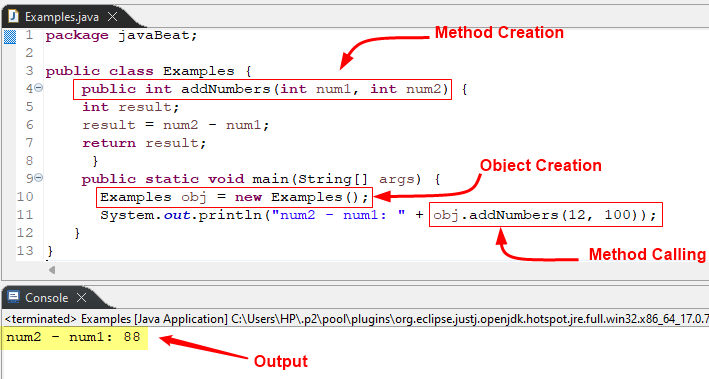
Example 3: How to Create and Call a Method Without Parameter List
In the following code, we create a user-defined method “findCube()” that doesn’t accept any parameter and doesn’t return anything:
package javaBeat;
import java.util.Scanner;
public class Examples {
void findCube()
{
System.out.print("Enter a Number: ");
Scanner scanObj = new Scanner(System.in);
Integer num1 = scanObj.nextInt();
Integer result;
result = (num1 * num1 * num1);
System.out.println("Square Root of Given Number: " + result);
scanObj.close();
}
public static void main(String[] args) {
Examples obj = new Examples();
obj.findCube();
}
}
In the findCube() method, we create an instance of the Scanner class and use it with the nextInt() method to get an integer from the user. After this, we use the “*” operator to find the cube of the specified number. Up next, we print the resultant cube on the console using the println(). In the main() method, we create an object of the class and call the findCube() method using that object:
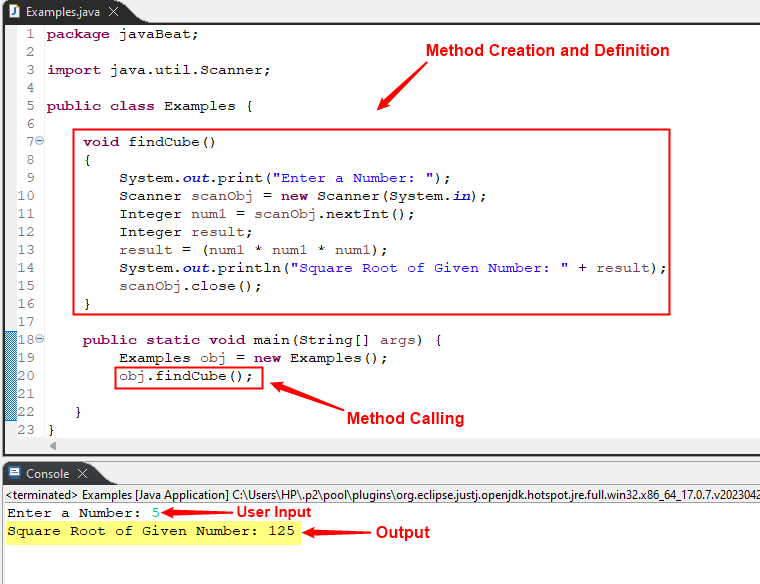
Example 4: How to Create and Call a Method of a Parent Class
Let’s create a parent class “Programmers” and a child class “JavaProgrammers”. In the parent class, create a method displaySaraly() to show the programmer’s salary:
package javaexamples;
public class Programmers {
int salary = 50000;
public void displaySalary() {
System.out.println("Programmers Salary: " + salary);
}
}
class JavaProgrammers extends Programmers {
int incentives = 15000;
public static void main(String args[]) {
JavaProgrammers obj = new JavaProgrammers();
obj.displaySalary();
System.out.println("Java Programmer's Bonus ⇒ " + obj.incentives);
System.out.println("Java Programmer's Gross Salary ⇒ " + (obj.salary + obj.incentives));
}
}
While creating the “JavaProgrammers” class, use the “extends” keyword to inherit the “Programmers” class. In the main() method, create an instance of the “JavaProgrammers” class and call the displaySalary() method of the “Programmers” class using that instance/object. Finally, use the println() method to show the Java programmer’s bonus and gross salary on the console:

This is how you can call a parent class method in Java. To learn more about it, follow our dedicated guide on how to call a Java method from another class.
Example 5: How to Create and Call an Abstract Method in Java
A method declared/created using the “abstract” keyword is known as an abstract method. It is declared in an abstract class while it is defined in another class(s). It can be public or protected, however, it cannot be created as private or final. Let’s declare an abstract method in the “CircumferenceofCircle” class and define and call it in the “Examples” class:
package javaexamples;
abstract class CircumferenceOfCircle {
abstract void showCircumference();
}
public class Examples extends CircumferenceOfCircle {
void showCircumference() {
int radius = 5;
Double pi = Math.PI;
System.out.println("The Circumference of a Circle ⇒ " + (2 *pi*radius));
}
public static void main(String args[]) {
CircumferenceOfCircle obj = new Examples();
obj.showCircumference();
}
}
In the above code, we create an abstract class “CircumferenceOfCircle” with an abstract method “showCircumference()”. We create another class Examples that extends the “CircumferenceOfCircle”. Within the Examples class, we define the showCricumference() method. Finally, we invoke the showCircumference() method from the main() method using a class object:

How to Call a Built-in Java Method
The methods already defined in the Java class libraries and ready to use are known as pre-defined, built-in, or standard methods. You can use these methods in your Java application directly by calling/invoking them using their name. The well-known examples of predefined methods include, “sqrt()”, “length()”, “max()”, “round()”, “equals()”, etc. Each of these methods belongs to a built-in Java class and serves a specific purpose. For example, when you call a built-in sqrt() method, it retrieves the square root of the provided value.
Example: Calling a Predefined Java Method
In the following example, we use several built-in methods to perform different mathematical operations on the input values:
package javaexamples;
public class Examples {
public static void main(String args[]) {
System.out.println("Sum: "+ Integer.sum(102, 72));
System.out.println("Absolute Value: "+ Math.abs(-123.2)); System.out.println("Maximum Number: "+ Math.max(212, 172));
System.out.println("Minimum Number: "+ Math.min(-112, 72));
System.out.println("Square Root: "+ Math.sqrt(121));
}
}
Here, first, we use the “sum()” method of the Integer class to compute the sum of given values. After this, we use the abs(), max(), min(), and sqrt() methods of the Math class to find the absolute value, maximum value, minimum value, and square root of the provided value:

That’s all about creating and calling methods in Java.
Final Thoughts
To create a method in Java, specify the access modifier, return type, and a valid name of the method, which is followed by a set of parentheses. Inside the parentheses, you may or may not provide the parameters list (if needed). To call/invoke a method, specify the method name followed by parenthesis and a semicolon. If a method is static, it can be called/accessed directly using the method name while if it is non-static, then it is called using the class instance. In this guide, we have explained all these concepts with appropriate examples.