A string is a sequence of characters; these characters can be alphabets/letters, symbols, or numbers. A string containing numbers is referred to as a numeric string. While manipulating the string data, you may encounter a situation where you need to check if a string is numeric or not. For example, when you take user input from a text field or text area, that input is always in the form of a string. In such cases, it is recommended to first check whether the input string is numeric and then proceed with the string manipulation accordingly.
How to Check if a String is Numeric Using Parsing?
Java provides several predefined parsing methods that can check if a string is numeric. These methods include parseInt(), parseFloat(), parseDouble(), parseLong(), and BigInteger(). Let’s discuss each of these methods with examples:
Method 1: Using Integer.parseInt()
Integer.parseInt() is a built-in Java method that accepts a decimal string as a parameter and parses it into an integer. You can implement the “parseInt()” method on the given string to check if it contains an integer. The following code will help you understand this method better:
public static boolean checkNumeric(String inputString){
boolean numericString;
if(inputString == null)
numericString = false;
else
{
try
{
Integer number = Integer.parseInt(inputString); numericString = true;
}
catch(NumberFormatException e)
{
numericString = false;
}
}
return numericString;
}
public static void main(String args[]) {
System.out.println("54321 is numeric: " + checkNumeric("54321"));
System.out.println("54 321 is numeric: " + checkNumeric("54 321"));
System.out.println("null is numeric: " + checkNumeric(null));
System.out.println("54321abc is numeric: " + checkNumeric("54321abc"));
System.out.println("54-abc is numeric: " + checkNumeric("54-abc"));
System.out.println("+54321 is numeric: " + checkNumeric("+54321"));
System.out.println("-54321 is numeric: " + checkNumeric("-54321"));
System.out.println("54.321 is numeric: " + checkNumeric("54.321"));
}
We call the user-defined “checkNumeric()” function from the main() method and pass the strings as its argument to check if they are numeric or not:

Method 2: Using Float.parseFloat()
parseFloat() is an inbuilt method of the Float class that can be used to parse a string into its equivalent floating point value. You can use the parseFloat() method to check if the given string contains numeric values. Here is a practical demonstration of this method:
Float number = Float.parseFloat(inputString);

Method 3: Using Double.parseDouble()
parseDouble() belongs to the “java.lang.Double” class that parses a numeric string into its equivalent double value. You can use this method to check whether the input string is a double-type numeric, as demonstrated in the following example:
Double number = Double.parseDouble(inputString);

Method 4: Using Long.parseLong()
This method parses the given string/character sequence into a signed long value. You can apply this method on any given string to check if it is a numeric string or not:
Long number = Long.parseLong(inputString);

Method 5: Using the new BigInteger()
The BigInteger class allows you to perform mathematical calculations on very big integers. You can use the BigInteger() constructor on the provided string to parse it into a BigInteger. Doing this will help you determine if the provided string is numeric or not:
BigInteger number = new BigInteger(inputString);
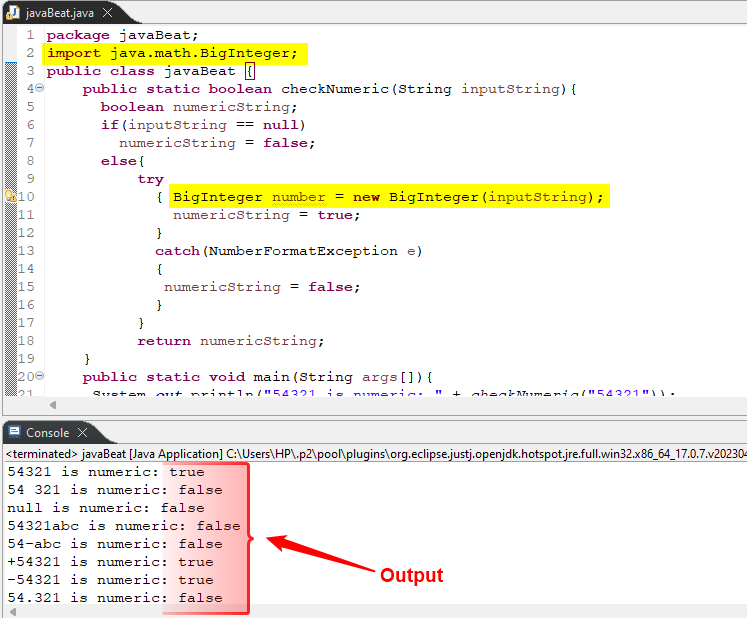
How to Check if a String is Numeric Using Apache Commons Java?
Apache Commons is an external library, therefore, to use it, first, it must be added to the “pom.xml” file of a Maven project, as follows:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.14.0</version>
</dependency>
pom.xml File:

Method 1: Using NumberUtils.isCreatable()
The NumberUtils class of Apache Commons Lang offers a static method “isCreatable()” that checks whether the input string is numeric. It returns true only if the input string is a valid number else it retrieves false. Also, it returns false in case the input string is empty, null, or blank:
package mavenExample;
import org.apache.commons.lang3.math.NumberUtils;
public class IsNumericString {
public static void main(String[] args) {
System.out.println("54321 is numeric: " + NumberUtils.isCreatable("54321"));
System.out.println("54 321 is numeric: " + NumberUtils.isCreatable("54 321"));
System.out.println("null is numeric: " + NumberUtils.isCreatable(null));
System.out.println("543abc is numeric: " + NumberUtils.isCreatable("543abc"));
System.out.println("54-abc is numeric: " + NumberUtils.isCreatable("54-abc"));
System.out.println("+54321 is numeric: " + NumberUtils.isCreatable("+54321"));
System.out.println("-54321 is numeric: " + NumberUtils.isCreatable("-54321"));
System.out.println("54.321 is numeric: " + NumberUtils.isCreatable("54.321"));
System.out.println("٣١٣٣ is numeric: " + NumberUtils.isCreatable("٣١٣٣"));
System.out.println("0XA12 is numeric: " + NumberUtils.isCreatable("0XA12"));
System.out.println("6.0220943E+23 is numeric: " + NumberUtils.isCreatable("6.0220943E+23"));
}
}

Method 2: Using NumberUtils.isParsable()
The NumberUtils class of Apache Commons offers a very convenient static method named “isParsable()”. It accepts a string as a parameter and checks if it’s parsable to a number or not. It returns true if the provided string is a number and it returns false if a string is null, blank, or contains whitespaces:
package mavenExample;
import org.apache.commons.lang3.math.NumberUtils;
public class IsNumericString {
public static void main(String[] args) {
System.out.println("54321 is numeric: " + NumberUtils.isParsable("54321"));
System.out.println("54 321 is numeric: " + NumberUtils.isParsable("54 321"));
System.out.println("null is numeric: " + NumberUtils.isParsable(null));
System.out.println("543abc is numeric: " + NumberUtils.isParsable("543abc"));
System.out.println("54-abc is numeric: " + NumberUtils.isParsable("54-abc"));
System.out.println("+54321 is numeric: " + NumberUtils.isParsable("+54321"));
System.out.println("-54321 is numeric: " + NumberUtils.isParsable("-54321"));
System.out.println("54.321 is numeric: " + NumberUtils.isParsable("54.321"));
System.out.println("٣١٣٣ is numeric: " + NumberUtils.isParsable("٣١٣٣"));
System.out.println("0XA12 is numeric: " + NumberUtils.isParsable("0XA12"));
System.out.println("6.0220943E+23 is numeric: " + NumberUtils.isParsable("6.0220943E+23"));
}
}

Method 3: Using StringUtils.isNumeric()
The isNumeric() method checks whether the provided string contains only Unicode digits. It retrieves “true” in the case of Unicode digits only. In case, the given string contains a Unicode symbol like “null”, “decimal point”, a “leading sign”, “whitespaces”, or an empty string, then it retrieves “false”.
The following code imports the StringUtils class from the Apache Commons and then uses the isNumeric() method on different strings to check if a string is numeric:
import org.apache.commons.lang3.StringUtils;
public class IsNumericString {
public static void main(String[] args) {
System.out.println("54321 is numeric: " + StringUtils.isNumeric("54321"));
System.out.println("54 321 is numeric: " + StringUtils.isNumeric("54 321"));
System.out.println("null is numeric: " + StringUtils.isNumeric(null));
System.out.println("54321abc is numeric: " + StringUtils.isNumeric("54321abc"));
System.out.println("54-abc is numeric: " + StringUtils.isNumeric("54-abc"));
System.out.println("+54321 is numeric: " + StringUtils.isNumeric("+54321"));
System.out.println("-54321 is numeric: " + StringUtils.isNumeric("-54321"));
System.out.println("54.321 is numeric: " + StringUtils.isNumeric("54.321"));
System.out.println("٣١٣٣ is numeric: " + StringUtils.isNumeric("٣١٣٣"));
}
}
The output signifies that the isNumeric() method returns true if the strings contain Unicode digits only. It returns false for all those strings that contain anything other than Unicode digits:

Method 4: Using StringUtils.isNumericSpace()
The isNumericSpace() is a bit more flexible method that returns “true” if the given string contains Unicode digits or whitespaces. In case, the given string contains a “null”, “decimal point”, a “leading sign”, or an empty string, then it retrieves “false”. The following example illustrates how the isNumericSpace() method works in Java:
import org.apache.commons.lang3.StringUtils;
public class IsNumericString {
public static void main(String[] args) {
System.out.println("54321 is numeric: " + StringUtils.isNumericSpace("54321"));
System.out.println("54 321 is numeric: " + StringUtils.isNumericSpace("54 321"));
System.out.println("null is numeric: " + StringUtils.isNumericSpace(null));
System.out.println("54321abc is numeric: " + StringUtils.isNumericSpace("54321abc"));
System.out.println("54-abc is numeric: " + StringUtils.isNumericSpace("54-abc"));
System.out.println("+54321 is numeric: " + StringUtils.isNumericSpace("+54321"));
System.out.println("-54321 is numeric: " + StringUtils.isNumericSpace("-54321"));
System.out.println("54.321 is numeric: " + StringUtils.isNumericSpace("54.321"));
System.out.println("٣١٣٣ is numeric: " + StringUtils.isNumericSpace("٣١٣٣"));
}
}
The output signifies that the isNumericSpace() method returns true if the strings contain Unicode digits or Unicode digits with whitespaces:

How to Check if a String is Numeric Using Regular Expressions?
Another convenient and flexible approach to checking numeric strings is regular expressions. To do this, create a search pattern and based on that pattern you can check whether a string is numeric. The following regexPattern checks if a string contains a valid number; if so, it returns true. It returns false for any string that contains a null value, alphabets, whitespaces, or a special character.
regexPattern = "-?\\d+(\\.\\d+)?";
Here, in the regex pattern:
- The “-?” symbol checks if the given string contains a minus sign (negative number).
- “\\d+” checks if the strings contain one or more digits.
- “(\\.\\d+)?” checks if the input string contains a decimal.
So, all in all, this regex pattern will return true for positive or negative integers, and positive or negative decimal numbers. Let’s implement this pattern to test its working on different strings:
public static boolean checkNumeric(String inputString)
{ boolean numericString;
String regexPattern = "-?\\d+(\\.\\d+)?";
if(inputString == null)
numericString = false;
else if(inputString.matches(regexPattern))
numericString = true;
else numericString = false;
return numericString;
}
Create a user-defined “checkNumeric()” function that accepts the input string as an argument. Within this function, create a regex pattern to test if the provided string is numeric or not. After this, compare the input string with the regex pattern using the if-else statements and return a boolean “true” or “false” accordingly. In the main() method, invoke the user-defined “checkNumeric()” function with different strings to check if they are numeric or not:
public static void main(String args[]) {
System.out.println("54321 is numeric: " + checkNumeric("54321"));
System.out.println("54 321 is numeric: " + checkNumeric("54 321"));
System.out.println("null is numeric: " + checkNumeric(null));
System.out.println("54321abc is numeric: " + checkNumeric("54321abc"));
System.out.println("54-abc is numeric: " + checkNumeric("54-abc"));
System.out.println("+54321 is numeric: " + checkNumeric("+54321"));
System.out.println("-54321 is numeric: " + checkNumeric("-54321"));
System.out.println("54.321 is numeric: " + checkNumeric("54.321"));
}
The strings that match the defined regex pattern result in a boolean true. On the other hand, the strings that don’t match the regex pattern result in a boolean false:
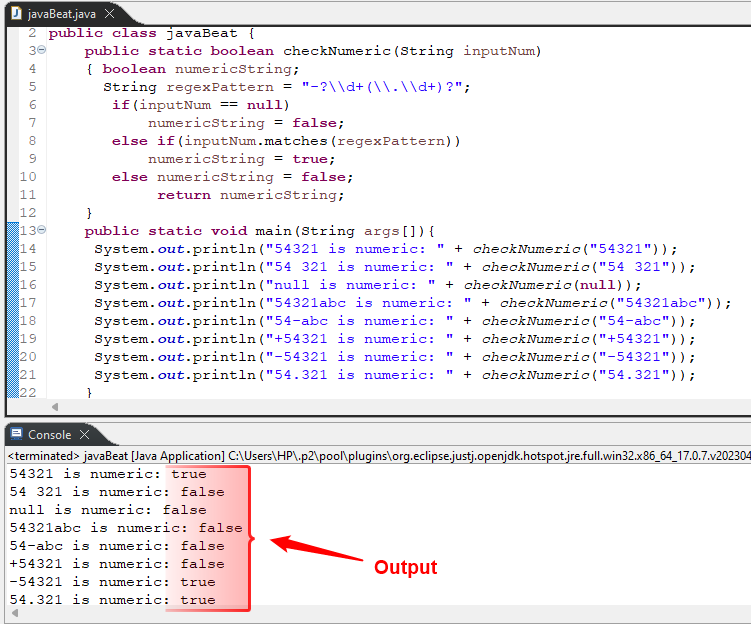
This is how you can check if the given string is numeric or not in Java.
Conclusion
Checking if a string is numeric helps you parse the given string and perform different numerical operations on it. You can do this using built-in parse methods, Apache Commons Lang, and regular expressions. Built-in parse methods are the most convenient way of checking if the string contains valid digits or not. Use these methods if you want to check a specific type of numeric string like integer, float, etc. Alternatively, you can use the Apache Common Lang to check the strings with more flexibility. However, if you want an all-in-one solution, then you can customize a regex pattern according to your preferences.