“ArrayIndexOutOfBoundsException” is a commonly faced error that occurs while working with the array data structure. As the name itself suggests, this error occurs when a Java user tries to access an array index outside the array’s valid bounds. When you encounter this error, the program crashes and produces unpredictable and unwanted results. Luckily! Java offers several solutions to fix the “Array Index Out Of Bounds” Exception.
This write-up will discuss all the possible reasons and suitable solutions to fix the stated error.
What Causes the ArrayIndexOutofBoundsException in Java
There might be several reasons that cause the “Array Index Out of Bounds” Exception in Java. The possible reasons along with respective output snippets are discussed below:
Reason 1: Exceeding Array Length
When a user tries to access an index that exceeds the maximum array bound, then an “ArrayOutOfBounds” Exception occurs in Java:
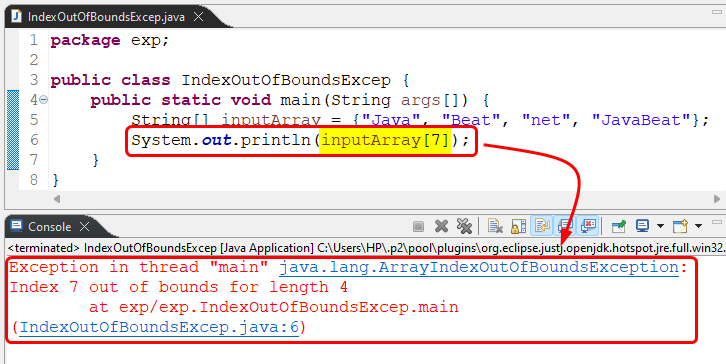
Reason 2: Accessing a Negative Index
Also, if a user accesses a negative index, the same error will arise in that case as well:

Reason 3: Exceeding an Index While Iterating Using a Loop
You may face the same error while executing a loop. It is such that the loop increments/decrements after each iteration. So if the loop condition is not specified appropriately, the “ArrayIndexOutOfBounds” exception occurs:
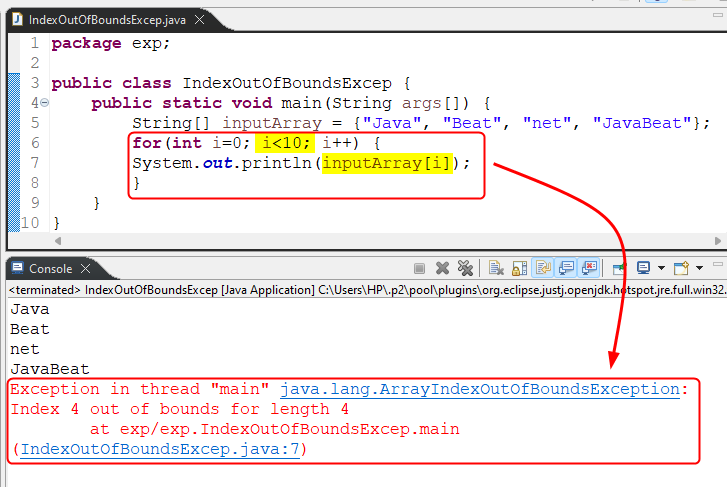
Reason 4: Accessing an Empty Array
In Java, an empty array has no element, so if you try to access an empty array you will encounter an ArrayIndexOutOfBounds exception:

How to Fix an “ArrayIndexOutOfBounds” Exception in Java
You can fix/resolve this error by employing one of the following solutions:
Solution 1: Validate the Array Length and Access the Index Accordingly
The most convenient solution to the “ArrayIndexOutOfBounds” exception is to validate the array’s length before accessing any of its indexes. For this purpose, you can use the length property to check the array’s total size and then access the array’s index accordingly:
package exp;
import java.util.Scanner;
public class IndexOutOfBoundsExcep {
public static void main(String args[]) {
String[] inputArray = { "Java", "Beat", "net", "JavaBeat" };
int i = inputArray.length;
System.out.println("Write a valid index between 0-"+(i-1));
Scanner scanInput = new Scanner(System.in);
int getIndex = scanInput.nextInt();
System.out.println("Array Element at Specified Index: " + inputArray[getIndex]);
scanInput.close();
}
}
In this code, we declare a string array and initialize it with different strings. Up next, we find the array size using the length property and print it on the console (i-1). After this, we get the array index to access using the Scanner class’ nextInt() method. Finally, we access the user-entered array index and print its respective array element on the console.
Output

Solution 2: Validate the Array Length and Execute the Loop Accordingly
In Java, using the “length” property in loops is a good practice to avoid the ArrayIndexOutOfBounds Exception. Doing this ensures that the loop iterates up to the last valid array index:
package exp;
public class IndexOutOfBoundsExcep {
public static void main(String args[]) {
String[] inputArray = { "Java", "Beat", "net", "JavaBeat" };
for(int i=0; i< inputArray.length; i++) {
System.out.println("Array Element at "+ i +" Index: " + inputArray[i]);
}
}
}
In this code, the loop starts iterating from the array’s 0th index and goes on till the array’s size/length. The use of the println() method ensures that each index value gets printed on the console appropriately:
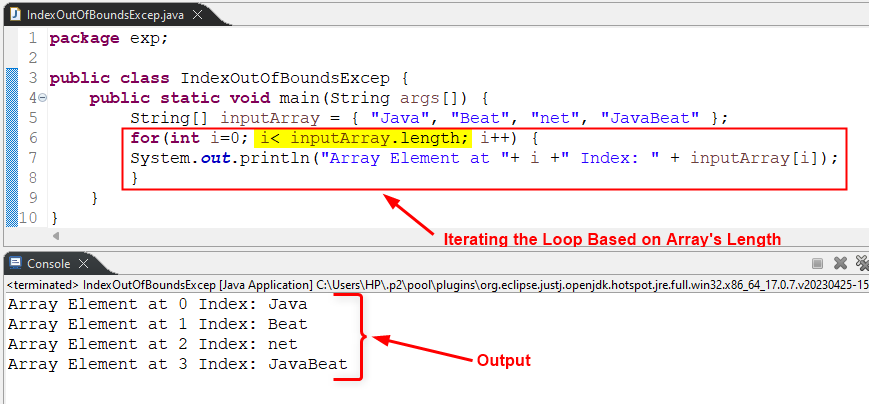
Solution 3: Use the Try-Catch Block
Another solution to the stated problem is the one that handles exceptions the best, i.e., the “try-catch” block. You can write the code that might cause the “ArrayIndexOutofBounds” exception in the try block and catch it in the catch block. Here is a demonstration of the stated problem:
package exp;
public class IndexOutOfBoundsExcep {
public static void main(String args[]) {
String[] inputArray = { "Java", "Beat", "net", "JavaBeat" };
try {
for(int i=0; i<= inputArray.length; i++) {
System.out.println("Array Element at "+ i +" Index: " + inputArray[i]);
}
}
catch(ArrayIndexOutOfBoundsException ex){
System.out.println("Index Exceeds the Array Size");
}
}
}
The loop successfully iterates from the 0th index till the array’s last valid index. Up next, when the loop exceeds the array’s length an exception occurs which is successfully handled by the “catch” block:

Solution 4: Use the For-Each Loop
Java’s for-each loop eliminates the array’s size headache. As it iterates through the entire array regardless of its size. So, using a for-each loop is one of the best possible solutions to avoid the “ArrayIndexOutOfBoundsException”:
package exp;
public class IndexOutOfBoundsExcep {
public static void main(String args[]) {
String[] inputArray = { "Java", "Beat", "net", "JavaBeat" };
for (String i : inputArray) {
System.out.println(i);
}
}
}
The for-each loop traverses the entire array and within the loop’s body, the println() method prints each traversed array element:

Solution 5: Create a Circular Array
A circular array is one where each element is connected to the next, up till the last element connects back to the first. You can create a circular array using the modulo operator “%” and iterate it using a loop. Doing this will eliminate the possibility of ArrayIndexOutOfBoundsException:
package exp;
public class IndexOutOfBoundsExcep {
public static void main(String args[]) {
String[] inputArray = { "Java", "Beat", "net", "JavaBeat" };
int arraySize = inputArray.length;
for (int i = 2; i <= 12; i++) {
System.out.println(inputArray[i%arraySize]); }
}
}
In this code, first, we compute the array size using the length property. After this, we use the for loop to traverse the given array according to the specified criteria. Within the loop, we utilize the “%” operator that ensures that the given array is circular and hence loop iterates accordingly:

This is how you can fix the ArrayIndexOutOfBoundsException in Java.
Final Thoughts
The ArrayIndexOutOfBoundsException occurs in Java for various reasons, such as exceeding an array length, accessing a negative index, or accessing an empty array. You can fix this error by validating the array length before accessing its elements, using a try-catch block or a for-each loop. Also, you can create a circular array to avoid the stated error.
Among all the stated solutions, the “for each” or “enhanced for” loop is the most convenient and recommended option. It iterates through the entire array and hence eliminates the size headache.