String manipulation is an integral component in development as it builds the foundation for different algorithms and functions. For working with strings, it is crucial to get the length of a string to determine the memory consumption, input validation, array operations, string comparisons, etc. In Java, there are built-in methods and third-party libraries that can be used to determine the total length of a String. Let’s understand different approaches and implement multiple methods for getting the length of the String in Java.
Quick Outline
This article provides methods for getting the String length in Java:
- Method 1: Using the Length()
- Method 2: Using the Length Property
- Method 3: Using Apache Commons Library
- Method 4: Using the Guava Library
Method 1: String Length Using the Length()
One of the simplest approaches to determining the length of a string in Java is to use the primary built-in length() method provided by the String class. The length() method counts the total characters and returns the length in the numerical value. Note that the white spaces are also counted as the “characters” and hence added to the total length of the character. The length() method accepts no argument and returns a number as the length of the string.
To invoke the length() method, first create a string variable as shown in the main() method of the code given below. Inside the print statement, the length() method is invoked using the string variable “str”. The output is then printed on the console:
package stringLength;
public class stringLength {
public static void main(String[] args) {
String str="Welcome to JavaBeat";
// prints the length of the String Variable
System.out.println("The length of the string is " + str.length());
}
}
Output
The output is as follows:
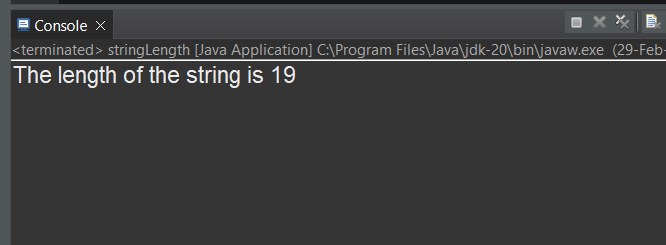
Method 2: String Array Length Using length
The String arrays store a fixed number of multiple strings at specific indexes in the memory. The length property gets the total size of an array. The length() method and the length property differ significantly.
In this code snippet, a string array “strArray” is created with different elements. The print statement displays the total length of the string as the strArray variable invokes the length property:
package stringLength;
public class stringLength {
public static void main(String[] args) {
String[ ] strArray= {"Welcome", "to", "JavaBeat"};
System.out.println("The length of the String Array is " + strArray.length); //prints the output
}
}
Output
The below screenshot shows the output of the above-mentioned code:
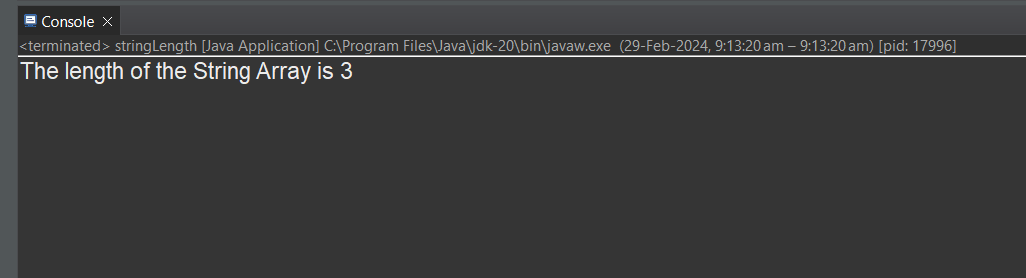
Comparison of Length() Vs Length Property
The following table demonstrates the comparison between the length() method and length property as both are often confused as similar:
Length() Method | Length Property |
---|---|
The length() method works for the strings and returns a numerical value. | The “length” property functions for the arrays and collections of objects. |
The String class length() method calculates the length of the string by counting the characters within the string. The numerical value is then returned by the method. | The “length” property counts the number of strings/elements at different indexes within the array. |
The length() method is used for the string variables. | .To get the total size of an array, the ‘length“ property is widely used |
Read more: Length vs Length() Method in Java
Method 3: String Length Using Apache Commons Library
The length of the string can also be determined by using the “StringUtils” class from the “Apache Commons Lang”. The StringUtils class provides different built-in static methods for working with the strings in Java.
To get the length of the string using the StringUtils class, add the following dependency to your pom.xml or build.gradle file of the Maven or Gradle project:
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.14.0</version>
</dependency>
</dependencies>
The dependency has been added successfully to the project:
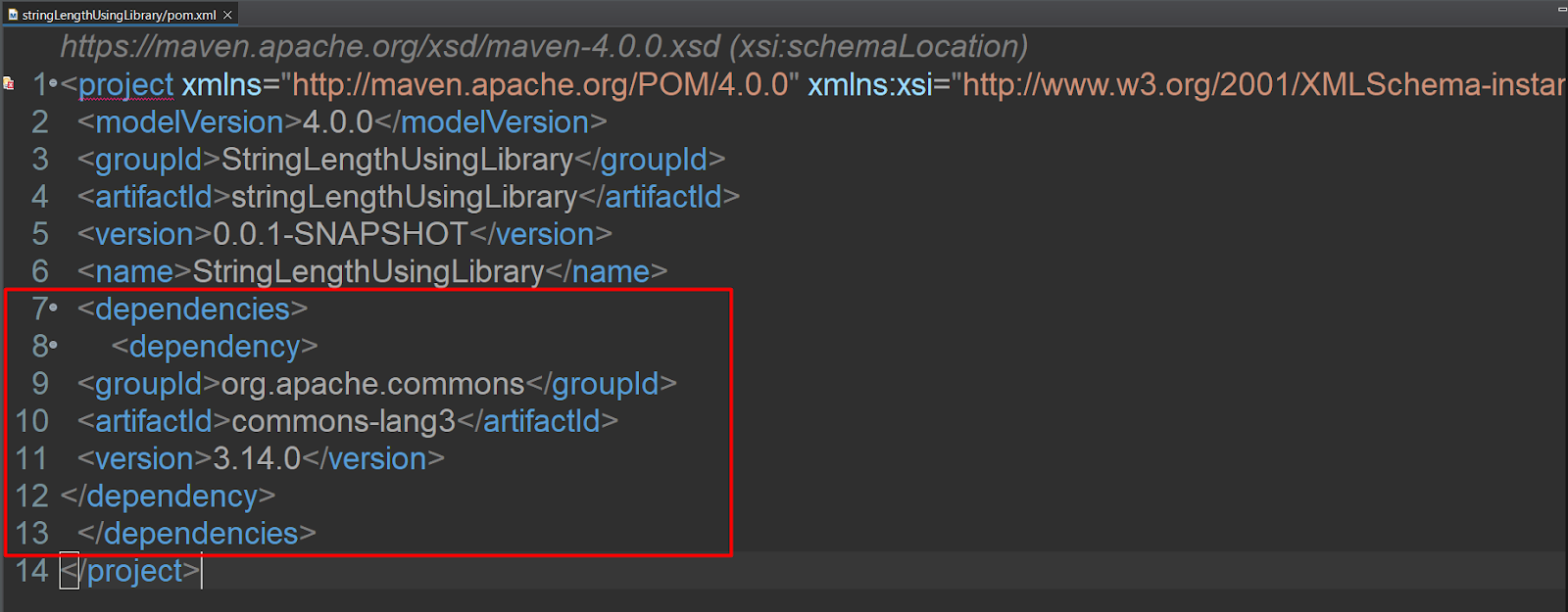
The StringUtils class is first imported from the “apache.commons.lang3” package using the given import statement. The string variable “str” is initialized with the given value within the main() method. Within the println() method, the StringUtils class invokes the static method length() that accepts the “str” as an argument. The length of the string is then printed:
package stringLengthUsingLibrary;
import org.apache.commons.lang3.StringUtils;
public class StringLengthUsingStringUtils {
public static void main(String[] args) //Main code inside the Main method {
String str="Welcome to JavaBeat"; //a string variable is created
System.out.println("String Length is " + StringUtils.length(str));
}
}
Output
The output is shown below:
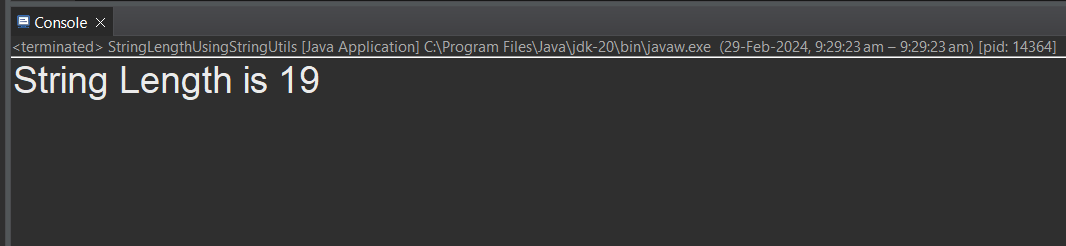
Method 4: String Length Using the Guava Library
Guava is an open-source core library provided by Google to write effective code and maximize the risk of errors. It contains different static methods for I/O operations, string manipulations, concurrency, etc.
The Guava Library requires the following dependency for working. Provide the given dependency to the pom.xml or build.gradle file of your project to include the functionality of the Guava library:
<dependencies>
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version> <!-- Use the latest version available -->
</dependency>
</dependencies>
The dependency has been added to the project:
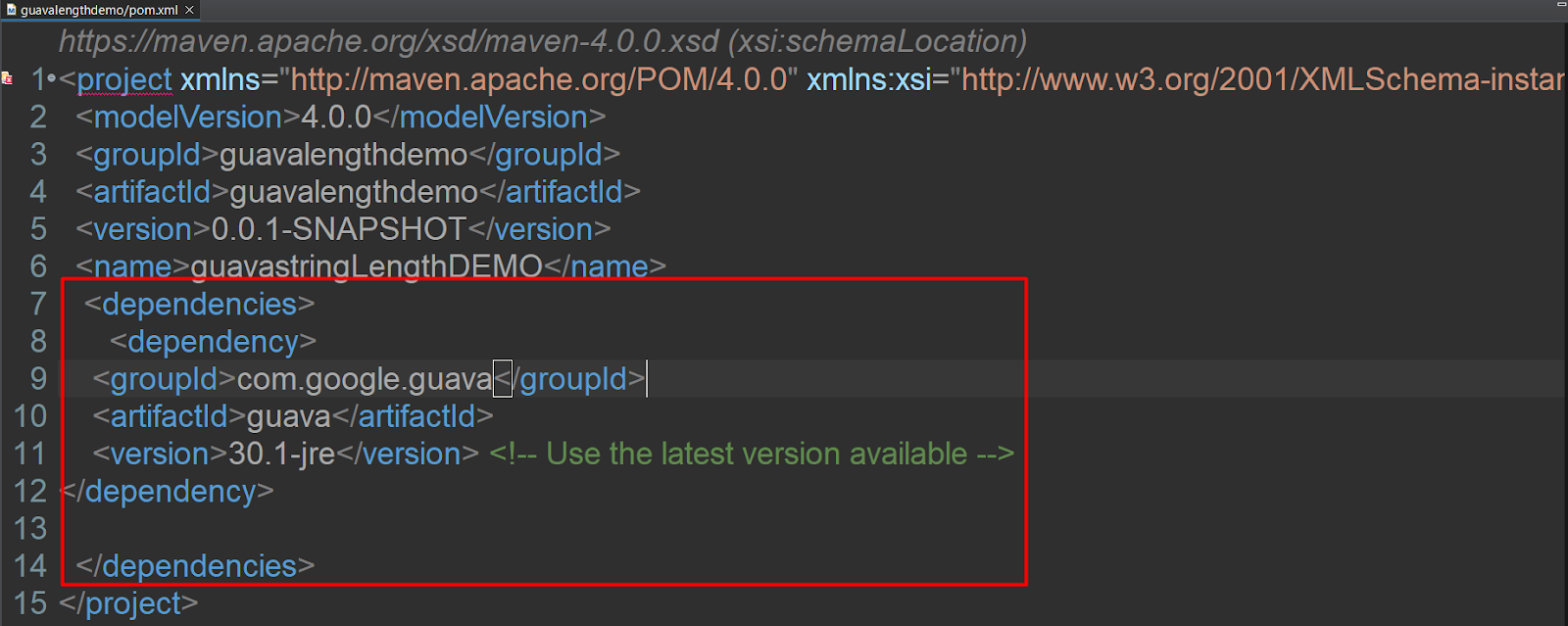
To work with the static functions of the Guava library, the given import statement includes the MoreObjects class from the google.common.base package. Inside the main() method, the string variable “str” is initialized with the given string. The MoreObjects class invokes the static method “firstNonNull()” that determines if the string is null or non-null. In case, if the provided string is null, it returns an empty string. On the other hand, it returns the total length of the string using the length() method which is then printed on the console:
package guavalengthdemo;
import com.google.common.base.MoreObjects;
public class guavalengthstring {
public static void main(String[] args) //Main method to Execute the code{
String str = "Welcome to JavaBeat"; //initializes a string
// Using Guava to get the length of the string
System.out.println("Length of the string: " + MoreObjects.firstNonNull(str, "").length());
}
}
Output
The output is given as follows:
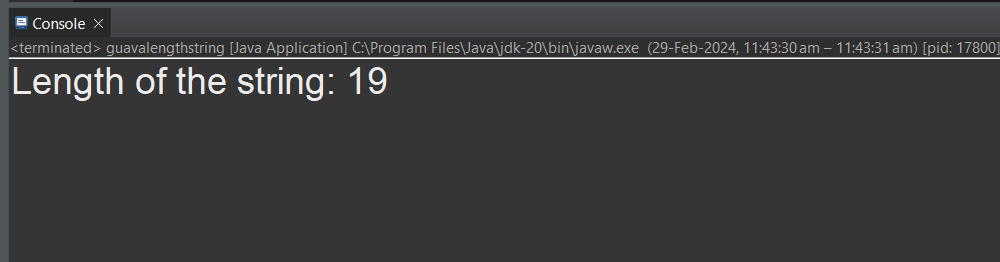
That is all from this guide.
Conclusion
In Java, working with strings is a common and crucial task for building applications. A string contains multiple characters in a sequence that are stored in the memory. For memory optimizations, increased performance, or string manipulation, it is important to get the length of a string. The length() method of the Java String and StringUtils class or the length property for Arrays are used to get the string length as shown in this article. This article provides different methods to determine the length of a string in Java.