Math is a predefined utility class in Java that belongs to Java’s default package named “java.lang”. It offers several methods that can be used with or without importing the Math class into a Java Program. These methods are used to perform different mathematical operations, such as computing absolute value, generating random numbers, computing the power of a value, and so on.
Quick Outline
This Java guide will explain all about importing the Math class using the following outlines:
- How Does Math Class Work in Java?
- Is Math Imported By Default in Java?
- How to Access/Use Java’s Math Class Without Importing it?
- What is the Need For Importing Java’s Math Class?
- How Do You Import Math in Java?
- Final Thoughts
How Does Math Class Work in Java?
The in-built classes in Java can be static or non-static. The static classes can be used without importing them into the Java program. On the other hand, the non-static classes must be imported first and then can be used anywhere in the program. The Math class is one of the Static classes that can be used without importing it into a program (via class name). Optionally, users can import it explicitly using Java’s “import” keyword.
The Math class offers numerous functions for performing mathematical calculations. The most significant among them are illustrated below:
Function | Description |
---|---|
Math.abs() | It retrieves an absolute value. |
Math.round() | It retrieves the given number by rounding it to the nearest/closest integer. |
Math.pow() | It computes the power of the specified number xy. |
Math.max() | It retrieves the largest/maximum among the given two values. |
Math.min() | It gives the smallest/minimum among the given two values. |
Math.sqrt() | It calculates the square root of the provided value. |
Math.random() | It generates a random number in the range (0 <= x < 1). |
Math.log() | It computes the logarithm of the given value. |
Math.floor() | It rounds down the provided value to the nearest integer. |
Math.ceil() | It rounds up the provided value to the nearest integer. |
Math.PI | It retrieves the value of 𝞹. |
Is Math Imported By Default in Java?
No, however, the package (i.e., java.lang) that contains the “Math” class is imported by default. Therefore, we can use/access this class without explicitly importing it.
How to Access/Use Java’s Math Class Without Importing it?
In Java, the methods and constants of the Math class can be used or accessed in any program using the dot syntax, i.e., “className.methodName()”.
Example: Use Math Functions Without Explicitly Importing It
In the following code, we will access several methods of the Math class without explicitly importing them:
package javaBeat;
public class javaBeat {
public static void main(String args[]) {
System.out.println("Absolute Value: " + Math.abs(-20.11));
System.out.println("Find Maximum: " + Math.max(12, 17)); System.out.println("Circumference of a Circle: " + 2 * Math.PI * 5); System.out.println("Generate a Random Value: " + Math.random());
}
}
In this code, the Math.abs(), Math.max(), Math.PI, and Math.random() functions are used to find an absolute value, a maximum value, the circumference of a circle, and a randomly generated value:
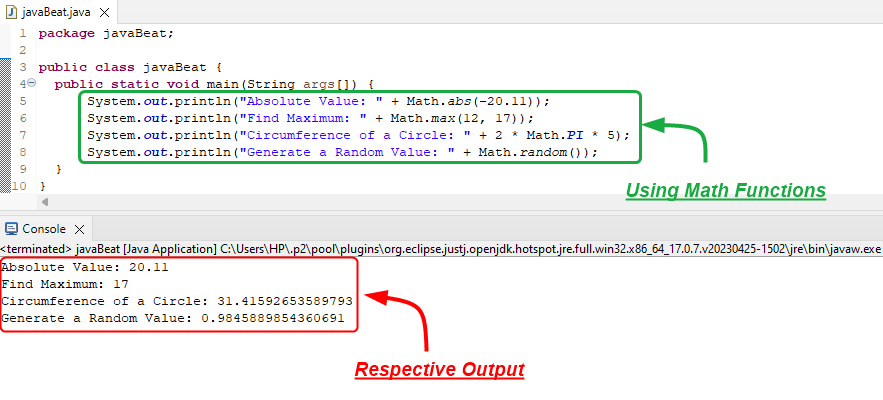
The math functions are working perfectly fine without explicitly importing the Math class.
What is the Need For Importing Java’s Math Class?
Importing the Math class helps us reduce the redundancy, avoids naming conflicts, and hence makes the code clean and easily readable. Once you import the Math class in your code, its variables and methods can be accessed anywhere in the code without using the class name.
How Do You Import Math in Java?
In Java, the entire Math class or only specific functions of the Math class can be imported using the “import” keyword. It is recommended to import the Math class statically so that its methods can be accessed and used without specifying the class name.
Syntax to Import Entire Math Class
Use “Math.*” to import all the methods and constants of Java’s Math class:
import static java.lang.Math.*;
Syntax to Import Only Specific Method of Math Class
Use function name instead of “*” to import only a specific member of the Math class:
import static java.lang.Math.functionName;
Example 1: How to Import Math Statically in Java?
In the following code, first, the Math class is imported statically, and after that, its different methods are accessed without using their class name:
package javaBeat;
import static java.lang.Math.*;
public class javaBeat {
public static void main(String args[]) {
System.out.println("Absolute Value: " + abs(-20.11));
System.out.println("Find Maximum: " + max(12, 17));
System.out.println("Find Minimum: " + min(12, 17));
System.out.println("Circumference of a Circle: " + 2 * PI * 5);
System.out.println("Generate a Random Value: " + random());
System.out.println("Find Power of a Number: " + pow(3, 3));
System.out.println("Find Square Root of 144: " + sqrt(144));
}
}

Each function gets executed successfully without using the Class name.
Example 2: How to Import a Specific Method of a Math Class in Java?
Suppose a user wants to compute the square root of five different values. For this purpose, he can import only the sqrt() function of the Math class instead of importing the entire class:
package javaBeat;
import static java.lang.Math.sqrt;
public class javaBeat {
public static void main(String args[]) {
System.out.println("Find Square Root of 144: " + sqrt(144));
System.out.println("Find Square Root of 121: " + sqrt(121));
System.out.println("Find Square Root of 49: " + sqrt(49));
System.out.println("Find Square Root of 72: " + sqrt(72));
System.out.println("Find Square Root of 84: " + sqrt(84));
}
}
In the above code, first, the sqrt() function is imported statically using the “java.lang.Math.sqrt” statement. After that, the imported method is invoked five times in the code to find the square root of 144, 121, 49, 72, and 84, respectively:
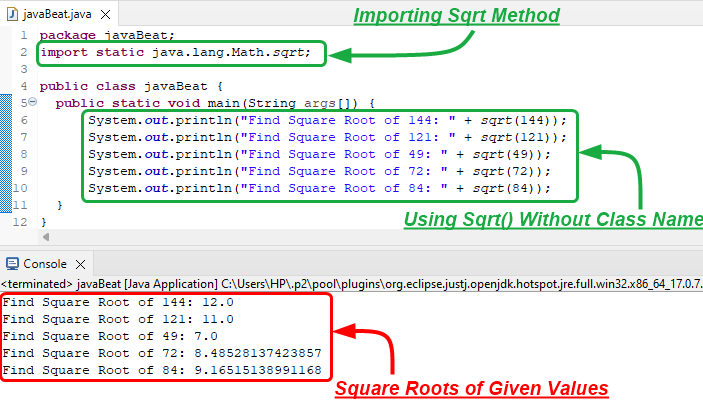
That’s All about importing Math in Java.
Final Thoughts
In Java, the Math class is not imported by default, however, the package to which it belongs (i.e., java.lang) is imported by default. Therefore, we can use/access this class without explicitly importing it. Importing the Math class can reduce the programmer’s efforts and ambiguity by avoiding naming conflicts, decreasing redundancy, and so on. To do that, use the “import static java.lang.Math.*;” statement at the start of your program. This Java blog has presented a comprehensive guide on importing the Math class using practical examples.