In Java, a pop() method is offered by a couple of classes (Stack and LinkedList) and an interface (Deque). It pops the top element from the respective data structure. More specifically, the pop() method of the “java.util.Stack” class removes the last inserted (top) element of the stack. However, if you apply the pop() method on an empty stack, you will encounter an EmptyStack exception.
Today’s guide will demonstrate what the pop() method is and how it works in Java. Also, this post will address what kind of exceptions you can face while working with the pop() method and how to fix them without any hassles.
What is the Stack.pop() in Java?
Stack is one of the Linear data structures in Java that works on the principle of LAST IN FIRST OUT (LIFO). To insert a new element into a stack, you can use the push() method while to remove it from the stack, use the pop() method. The pop() method pops and removes a single element at a time and that is always the topmost(last inserted) element of the stack. The working of the pop() method is demonstrated in the following figure:

How Does the Stack.pop() Method Work in Java?
The pop() method doesn’t accept any parameter and is implemented using the stack name. It gets the stack’s top element and removes it. To execute this function on a stack, use the syntax:
stackName.pop();
Replace the “stackName” with the given stack name (from which you want to remove an element).
Example 1: Pop/Remove a Single Element From a Stack
The following example uses the pop() method to pop/remove an element from a stack:
package javaexamples;
import java.util.*;
public class StackExample {
public static void main(String args[])
{
//creating a Stack
Stack<Integer> evenNum = new Stack<Integer>();
//Inserting elements
evenNum.push(2);
evenNum.push(4);
evenNum.push(6);
evenNum.push(8);
evenNum.push(10);
evenNum.push(12);
//Printing the Original Stack
System.out.println("Original Stack: " + evenNum);
//Popping Last Element
System.out.println("The Popped/Removed Element: " + evenNum.pop());
//Printing the Modified Stack
System.out.println("Modified Stack After Pop: " + evenNum);
}
}
In the above code, first, we create an empty stack and then insert six elements into it using the push() method. After this, we use the “pop()” to delete the last inserted stack element. Finally, we print the original and modified stack on the console:

Example 2: Pop Multiple Elements From a Stack
If you want to pop multiple elements from a stack, you can use the pop() method as often as the elements you want to pop. Or alternatively, you can use the pop() method within a loop. For example, the following code pops the top three elements from the stack:
package javaexamples;
import java.util.*;
public class StackExample {
public static void main(String args[])
{
//creating a Stack
Stack<Integer> evenNum = new Stack<Integer>();
//Inserting elements
evenNum.push(2);
evenNum.push(4);
evenNum.push(6);
evenNum.push(8);
evenNum.push(10);
evenNum.push(12);
// Printing the Original Stack
System.out.println("Original Stack: " + evenNum);
// Popping Top 3 Elements
for(int i=0; i<3; i++) {
System.out.println("The Popped Element: " + evenNum.pop());
}
// Printing the Modified Stack
System.out.println("Modified Stack After Pop: " + evenNum);
}
}
We use a for loop to iterate over the given stack. Within the loop, we use the pop() method on the “evenNum” stack to remove the top three elements from it:

Example 3: How to Pop All Elements of a Stack?
To pop all elements of the stack, use a loop and specify its total size as the termination condition of the loop:
import java.util.*;
public class StackExample {
public static void main(String args[])
{
Stack<Integer> evenNum = new Stack<Integer>();
evenNum.push(2);
evenNum.push(4);
evenNum.push(6);
evenNum.push(8);
evenNum.push(10);
evenNum.push(12);
// Printing the Original Stack
System.out.println("Original Stack: " + evenNum);
//finding total size of the stack
int stackSize = evenNum.size();
// Popping all Elements
for(int i=0; i< stackSize; i++) {
System.out.println("The Popped Element: " + evenNum.pop());
}
// Printing the Modified Stack
System.out.println("Modified Stack After Pop: " + evenNum);
}
}
All the code is the same as in the previous example, the only difference is the loop’s termination criteria. We find the stack’s total size using the size() method and specify it as the loop’s termination condition:
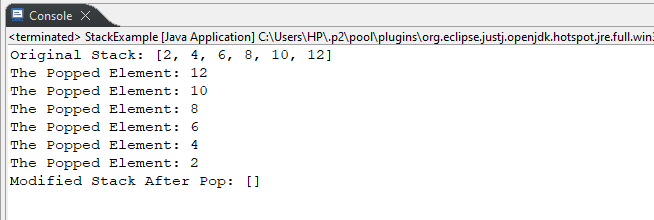
All the elements have been successfully popped.
Example 4: How to Pop a Middle Element of a Stack?
You can use the conditional statements to determine the stack’s middle element. The middle element will be popped according to the stack size. The given stack can be either even-sized or odd-sized. Let’s learn how to find and pop the middle element of a stack:
package javaexamples;
import java.util.*;
public class StackExample {
public static void main(String args[]) {
Stack<Integer> evenNum = new Stack<Integer>();
evenNum.push(2);
evenNum.push(4);
evenNum.push(6);
evenNum.push(8);
evenNum.push(10);
evenNum.push(12);
// Printing the Original Stack
System.out.println("Original Stack: " + evenNum);
//Declaring a New Stack
Stack<Integer> newStack = new Stack<Integer>();
//adding elements of evenNum stack into newStack
while (!evenNum.empty()) {
newStack.add(evenNum.pop());
}
//Finding Stack Size
int size = newStack.size();
//finding Middle Element
if (size % 2 == 0) {
//for even-size stack
int middleElement = (size / 2);
for (int i = 0; i < size; i++) {
//skipping the middle element
if (i == middleElement)
continue;
evenNum.push(newStack.get(i));
}
} else {
//for odd-size stack
int middleElement = (int) Math.ceil(size / 2);
for (int i = 0; i < size; i++) {
//skipping the middle element
if (i == middleElement)
continue;
evenNum.push(newStack.get(i));
}
}
//displaying the modified stack
System.out.print("Printing Modified Stack: [");
while (!evenNum.empty()) {
Integer stackElement = evenNum.pop();
System.out.print(stackElement + " ");
}
System.out.print("]");
}
}
- Initially, we create an empty stack “evenNum” and then we add six even numbers into it using the push() method.
- After this, we create a new stack “newStack” and initialize it with the elements of the “evenNum” stack (in reverse order).
- Up next, we use the “size()” method to determine the stack size (i.e., even-sized stack or odd-sized stack).
- If the stack is even-sized, we skip the middle element and push the remaining elements from newStack to the original stack.
- If the stack is odd-sized, we use the “Math.ceil()” method to round up and skip the element accordingly.
- Finally, we print the modified stack on the console:

How to Fix an EmptyStackException?
Suppose you apply the pop() method on an empty stack. In that case, you will encounter the following exception:
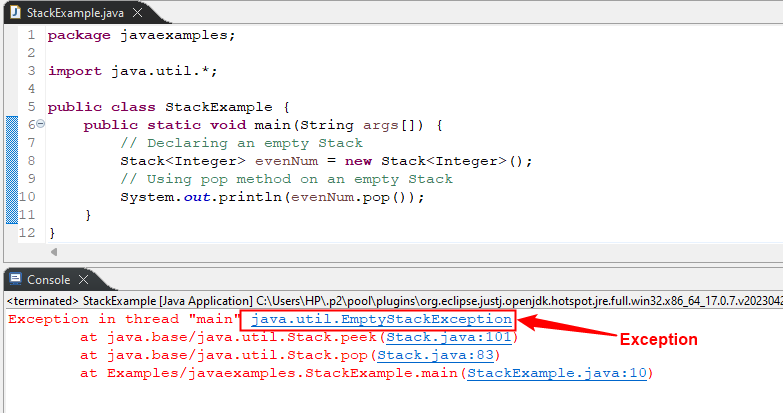
To avoid this exception, it is recommended to check if the given stack is empty and then pop the stack element accordingly. Here is a practical demonstration of this concept:
package javaexamples;
import java.util.*;
public class StackExample {
public static void main(String args[]) {
Stack<Integer> evenNum = new Stack<Integer>();
if (evenNum.isEmpty()) {
System.out.println("Given Stack is Empty");
}
else {
System.out.println("The Removed Element is " + evenNum.pop());
}
}
}
In the above code, we use the isEmpty() method in the if statement that checks if the given stack is empty. If yes, we will get a message “Given stack is empty” instead of an exception. However, if the given stack contains some elements, the top element will be removed from it using the pop() method:

That’s all about the Stack.pop() method in Java.
Conclusion
The stack is a Linear data structure in Java that works on the “LIFO” principle. It offers a pop() method that pops/removes the last entered element from the stack. It doesn’t accept any parameters. When you apply the pop() method on a stack, it retrieves the topmost/last inserted element of the stack and deletes it. If you implement this method on an empty stack, you will face an exception “java.util.EmptyStackException”. In this guide, we have illustrated various use cases of the Stack.pop() method in Java.