A set in Java is an unordered/unsorted collection of objects that contains only unique elements. While working with a set data structure, developers often check the existence of an element before performing any specific operation. This practice helps them avoid unexpected and unwanted circumstances. For this purpose, the set contains() method is used in Java.
This Java blog will help you check the presence/existence of any specific element in a set via the contains() method.
How to Use Set contains() Method in Java
The set contains() method accepts the target element as a parameter and is used with a dot syntax:
setName.contains(Object targetElement);
Here,
- “setName” represents a target set (that may or may not contain the target element).
- “targetElement” represents an element whose existence will be checked/tested in the specified set.
Return Value: “True” (if the element exists/found in the set) and “False” (if the element is not found).
Example 1: Using Set contains() Method in Java
The below example demonstrates the working of the set “contains()” method in Java:
import java.util.*;
public class SetContainsExample {
public static void main(String[] args) {
Set<String> empData = new HashSet<String>();
empData.add("Joseph");
empData.add("27");
empData.add("New York");
empData.add("Author");
empData.add("javabeat.net");
System.out.println("Original Set Elements ==> " + empData);
System.out.println("\nSet contains 'John'? " + empData.contains("John"));
System.out.println("Set contains 'Joseph'? " + empData.contains("Joseph"));
System.out.println("Set contains '27'? " + empData.contains("27"));
System.out.println("Set contains '30'? " + empData.contains("30"));
System.out.println("Set contains '.net'? " + empData.contains(".net"));
System.out.println("Set contains 'New York'? " + empData.contains("New York"));
System.out.println("Set contains 'Author'? " + empData.contains("Author"));
System.out.println("Set contains 'javabeat.net'? " + empData.contains("javabeat.net"));
}
}
In the above-given code block:
- We create a set “empData” of type “string” and initialize it with different string elements.
- Up next, we invoke the “contains()” method on the set “empData” to check the existence of different elements.
- Finally, we wrap the contains() method within the println() method to print the respective output on the console:
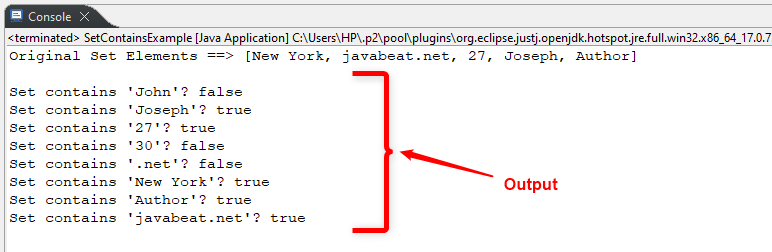
Example 2: Removing null Values From a Set
Let’s learn how to use the contains() method to check and remove a null value from a set:
import java.util.*;
public class SetContainsExample {
public static void main(String[] args) {
Set<String> empData = new HashSet<String>();
empData.add("Joseph");
empData.add("27");
empData.add("New York");
empData.add(null);
empData.add("Author");
empData.add("javabeat.net");
if(empData.contains(null))
{
System.out.println("Set Contains a Null Value ==> " + empData);
empData.remove(null);
System.out.println("Null Removed! Modified Set is ==> " + empData);
}
else
{
System.out.println("Set Does Not Contains a Null Value ==> "+ empData);
}
}
}
In the above code,
- We use the “contains()” method within the if statement to check the presence of a null value in the given set.
- Within the if block, we invoke the remove() method on the given set to remove the null value from it. Also, we execute the println() method to print the actual and modified set on the console.
- The else block executes if the set doesn’t contain a null value:

Till now, we have illustrated how you can check the existence of a specific element within a set. But what if you have to compare the entire set with another set? Well! In that case, you can utilize the containsAll() method instead of the simple “contains()” method. The containsAll() compares the given sets and retrieves true if and only if all elements are the same in both sets.
Example 3: Using Set containsAll() Method in Java
In this code, we will be using the containsAll() method to compare all the elements of two given sets:
import java.util.*;
public class SetContainsExample {
public static void main(String[] args) {
Set<String> empData1 = new HashSet<String>();
Set<String> empData2 = new HashSet<String>();
empData1.add("Joseph");
empData1.add("27");
empData1.add("New York");
empData2.add("Joseph");
empData2.add("27");
empData2.add("New York");
if(empData1.containsAll(empData2))
{
System.out.println("All Elements of the Given Sets are Same");
}
else
{
System.out.println("All(or Some) Elements of the Given Sets are Not Same");
}
}
}
In this code,
- We have two string-type sets: “empData1” and “empData2”.
- After this, we invoke the containsAll() method on the given sets which will return a boolean “true” or “false”.
- We use the containsAll() method within the if-else statement to print a custom message based on the retrieved output:

This sums up the use of the Set contains() method in Java.
Final Thoughts
To use the set contains() method in Java, pass the elements to be checked in it as an argument. As a result, the contains() method retrieves one of two boolean values: “true” or “false”. The boolean true ensures that the specified element exists in the target set. On the other hand, the false value indicates that the specified element doesn’t exist in the selected set. If you are supposed to check the existence of all elements of one set into another, then use the containsAll() method instead. This guide has illustrated the use of the Set contains() method with different examples.