In Java, programmers often need to add and store records in a container. The “Array” is one such container that efficiently stores the values of the same type and uses the memory in an optimum manner. Moreover, it reduces the code complexity i.e., no involvement of library or a class. This makes the “Array” a user-friendly approach with simpler syntax and faster implementation.
Java does not contain an in-built method for adding an element(s) to an array like other programming languages. The reason is that the array is of fixed size. However, there are some alternate approaches that add a single as well as multiple elements to an array.
Contents Overview
- What is an Array in Java?
- Why “Array” Should be Considered For Adding an Element in Java?
- Demonstration of Adding an Element to an Array in Java
- Utilized Common Methods
- How to Add an Element to an Array in Java?
What is an Array in Java?
“Array” in Java is of fixed size that contains the data in a sequence. It is utilized to contain various values in a single variable instead of assigning individual variables for each value.
Syntax
int[] array= new int[5];
int[] array = {1, 2, 3, 4, 5, 6};
The first syntax allocates the size i.e., “5” of the array whereas in the second syntax, there is no size assigned but the values are stored immediately.
Note: The index starts from “0” in the array.
Why “Array” Should be Considered For Adding an Element in Java?
There are multiple functionalities or classes in which the elements can be added. An “array”, however, is preferred or should be considered due to the fact that invoking an element in the array is convenient via indexing which makes the search procedure easy. Moreover, in the process of storing multiple values of the same type, “Arrays” come in handy. Therefore, adding an element or multiple elements to an “array” should be considered in Java.
Demonstration of Adding an Element to an Array in Java
Below is the demonstration in which the difference in the array can be seen before and after adding an element to the array:

Utilized Common Methods
Below are the common methods used for adding an element(s) to an array in the examples:
Arrays.toString(): This method retrieves a string representation against the target array’s content.
Syntax
public static String toString(int[] array)
In this syntax, “array” refers to the array whose string representation is to be retrieved.
Note: The array can also be of “byte”, “double”, “float”, “char”, “Object”, “long”, “short”, and “boolean” types.
Arrays.asList(): This method gives a fixed-sized list against the given array.
Syntax
public static List asList(T... array)
In the given syntax, “array” represents the array to be converted into a list.
toArray(): This method gives an array containing all the elements in an ArrayList.
Syntax
arraylist.toArray(T[] array)
Here, “T” refers to the array’s type, and “T[ ] array” represents an array where ArrayList elements are contained.
How to Add an Element to an Array in Java?
An element(s) can be added to an array via the following approaches:
- Creating a new Array.
- ArrayList.
- “System.arrayCopy()” Method.
Approach 1: Add an Element to an Array by Creating a New Array
In this approach, single as well as multiple elements can be added to an array with the help of a newly created array with a size greater than the already created array.
Example 1: Add a Single Element to an Array by Creating a New Array
This example adds a single element to an array using a newly created array of length “1” greater than the already created array:
package jbArticles;
import java.util.Arrays;
public class AddElem {
public static void main(String[] args) {
int[] array = {1, 2, 3};
int len = array.length;
int[] separateArray = new int[len+1];
int addValue = 4;
System.out.println("Before Adding Element to Array -> "+Arrays.toString(array));
for(int i = 0; i < len; i++) {
separateArray[i] = array[i];
}
separateArray[len] = addValue;
System.out.println("After Adding Element to Array -> "+Arrays.toString(separateArray));
}}
Code Explanation
- First, import the specified library to make use of arrays.
- Define an integer array with the given values and fetch its length via the “length” property.
- Now, initialize a new array having the length “1” greater than the previously created array in order to add a single element along with the other array(former) elements.
- Initialize an integer value that represents the element to be added to the new array.
- Apply the “for” loop that traverses through the first array’s length and allocates the new array the elements of the previously created array.
- At the last index specified as “separateArray[len]” in the code, append the initialized integer value such that a single element is added to the array and lastly, return the new array.
Output

In this output, it can be clearly seen that the new initialized integer is added to the newly created array.
Example 2: Adding Multiple Elements to an Array by Creating a New Array
In the following demonstration, multiple elements can be added to an array via a newly created array:
package jbArticles;
import java.util.Arrays;
public class AddElem {
public static void main(String[] args) {
int[] array = {1, 2, 3};
int len = array.length;
int[] separateArray = new int[len+2];
int addValue = 4;
int addValue2 = 5;
System.out.println("Before Adding Element to Array -> "+Arrays.toString(array));
for(int i = 0; i < len; i++) {
separateArray[i] = array[i];
}
separateArray[len] = addValue;
separateArray[len+1] = addValue2;
System.out.println("After Adding Elements to Array -> "+Arrays.toString(separateArray));
}}
Code Explanation
- Repeat the steps for creating a default array and returning its length.
- Now, create a new array having the size “2” greater than the default array to add 2 elements to the array.
- Initialize the 2 integers to be added.
- Moving ahead, similarly, apply the “for” loop to assign the former array elements to the new array.
- Finally, assign the initialized integer values to the last two indices of the new array and display the updated new array.
Output

As analyzed, the two defined integer values are added to the new array appropriately.
Example 3: Add an Element to an Array at a Target Index by Creating a New Array
A new array can also be used to add an element to the array at a target index via the following demonstration:
package jbArticles;
import java.util.Arrays;
public class AddElem {
public static void main(String[] args) {
Integer array[] = {1, 2, 3};
int len = array.length;
int index = 2;
System.out.println("Before Adding Element to Array -> "+Arrays.toString(array));
Integer separateArray[] = new Integer[len + 1];
int j = 0;
for(int i = 0; i<separateArray.length; i++) {
if(i == index) {
separateArray[i] = 25;
} else {
separateArray[i] = array[j];
j++;
}}
System.out.println("After Adding Element to Array at index (2)-> "+Arrays.toString(separateArray));
}}
Code Explanation
- Recall the steps for defining an array and retrieving its length.
- Now, specify/define the index at which a new element/item is to be added/appended.
- Create a new integer array using the “Integer” class having the size “1” greater than the formerly defined array.
- Apply the “for” loop such that at the specified index, the value “25” becomes appended to the new array and the remaining values at the remaining indices.
Output

Here, it can be seen that the specified element is inserted at the target index appropriately.
Approach 2: Add an Element to an Array Using “ArrayList”
An element can also be added to an array using the “ArrayList” class via the following steps:
- Append the created array to an ArrayList.
- Add an element to ArrayList.
- Convert the List to Array via the “toArray()” Method.
Below is the example code:
package jbArticles;
import java.util.Arrays;
import java.util.ArrayList;
public class AddElem {
public static void main(String[] args) {
Integer[] array = {1, 2, 3};
System.out.println("Before Adding Element to Array -> "+ Arrays.toString(array));
ArrayList<Integer> list = new ArrayList<Integer>(Arrays.asList(array));
list.add(4);
array = list.toArray(array);
System.out.println("After Adding Element to Array -> "+ Arrays.toString(array));
}}
Code Explanation
- Define an integer array and return its string representation via the “Arrays.toString()” method.
- Now, create an ArrayList of “int” type via the “Integer” class that converts the defined array previously to the list.
- Add the given integer to the ArrayList using the “add()” method.
- Convert the “List” again to “Array” and get the arrays’ string representation.
Output

As seen, the stated integer is added to the array successfully.
However, to add multiple elements to an array using ArrayList, apply the “add()” method as per the requirements repeatedly:
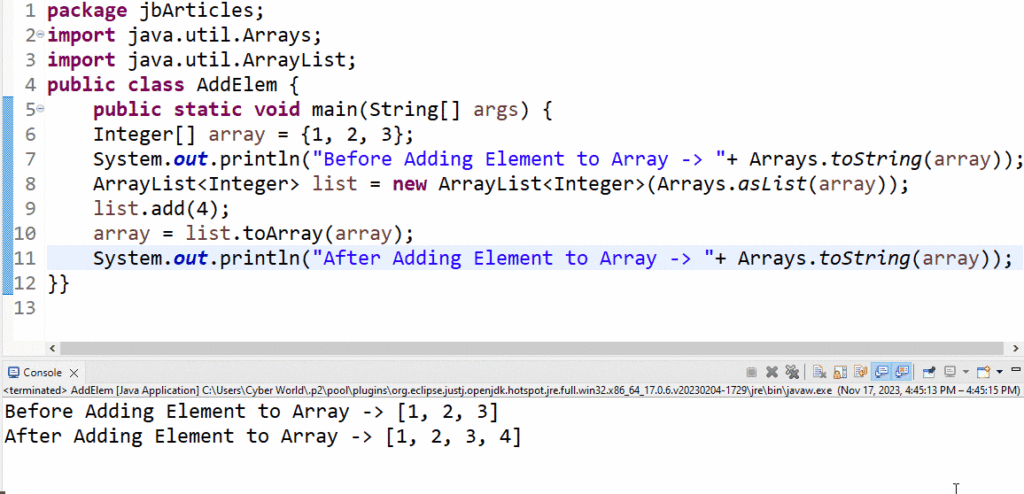
Approach 3: Add an Element to an Array Using the “System.arrayCopy()” Method
This method copies a source array, at a target start position, to a destination at the particular index. It can be applied to make a copy of the array and add element(s) to it.
Syntax
public static void arraycopy(Object source, int sourceind, Object des, int destind, int length)
Syntax Explanation
- “source” represents the source array.
- “sourceind” indicates the source array’s start index.
- “des” refers to the final/destination array.
- “destind” points to the beginning index of the destination array.
- “length” corresponds to the elements to be copied from the source to the destination array.
Now, proceed to the following code that adds an element to the array:
package jbArticles;
import java.util.Arrays;
public class AddElem {
private static Integer[] addElement(Integer[] newArray, int value) {
Integer[] array = new Integer[newArray.length + 1];
System.arraycopy(newArray, 0, array, 0, newArray.length);
array[newArray.length] = value;
return array;
}
public static void main(String[] args) {
Integer[] givenArray = {1, 2, 3};
System.out.println("Before Adding Element to Array -> " + Arrays.toString(givenArray));
givenArray = addElement(givenArray, 4);
System.out.println("After Adding Element to Array -> " + Arrays.toString(givenArray));
}}
Code Explanation
- Declare the function “addElement()” having the return type as an integer array that contains the two parameters.
- The first parameter indicates the array to be passed and the second parameter represents the number of elements to be added to a new copy of an array.
- In the function definition, create an array of length “1” greater than the passed array.
- Apply the “System.arraycopy()” method to copy from the passed array to the new array from the start such that all the elements from the passed array are copied to this array indicated by the fetched length.
- Add the “4” value to the last index of the array.
- In “main”, declare an integer array and return it as a string via the “toString()” method.
- Invoke the “addElement()” function by passing the array and the number of elements i.e., “4” to be added to the array and get the array as a string.
Output

The “4th” element is added to the copied array successfully.
Likewise, in this case, multiple elements can be added to the copied array by modifying the function parameters and increasing the array size, as demonstrated below:

Conclusion
In Java, an element(s) cannot be added to an array directly. However, there are some alternative approaches to do so such as creating a new array, using “ArrayList” or via the “System.arrayCopy()” method. However, multiple elements can also be added in all three approaches by modifying the applied method and increasing the array size.