Enums are a part of many programming languages including Java. An enum can be declared/created using a fixed set of constant values. The values specified in the enum can not be changed or modified. The enums are implemented in the code according to the need. To work with enums effectively, different built-in methods are used in Java. One such method is valueOf() which will be discussed in great detail in this blog.
How to Implement Enums in Java?
The enums in Java are declared using the “enum” keyword along with the specific identifier and afterward, the values are declared to those enums. Enums consist of fixed values. There are multiple methods of enum in Java that include values(), valueOf(), toString(), etc.
Note: To find more about enums in Java, read this dedicated guide.
In this article, we will implement the valueOf() method of enum in Java using practical examples.
Enum valueOf() Method in Java
The valueOf() method of the enum in Java returns the specified value from the declared enums. It is implemented with multiple methods of the enum like the ordinal() to fetch the index of the enum, and the values() method that returns all the declared values in the enum. The syntax of the valueOf() method is implemented below.
public static <T extends Enum<T>> T valueOf(Class<T> enumType, String name)
- T is the enum type.
- enumType returns a constant for the class object.
- The “name” is a return parameter for the constant to be returned.
Now, we will move ahead to implement the code examples of the valueOf() method in Java.
Example 1: Basic Implementation of the ValueOf() Method in Java
Let’s implement the ValueOf() method in Java to see how it works.
// Demonstration of the Example USing enums
enum Languages { Python, Javascript, Java, Ruby;
}
//Declare the Class
class ValueOfExample1 {
public static void main(String args[]) {
Languages p1 = Languages.valueOf("Python");
Languages p2 = Languages.valueOf("Javascript"); Languages p3 = Languages.valueOf("Java");
Languages p4 = Languages.valueOf("Ruby");
System.out.println("The Output is : ");
//Return the value
System.out.println("Languages.valueOf(Python) " + " " + p1.toString());
System.out.println("Languages.valueOf(Javascript) " + " " + p2.toString());
System.out.println("Languages.valueOf(Java) " + " " + p3.toString());
System.out.println("Languages.valueOf(Ruby) " + " " + p4.toString());
}
}
In the above code block:
- The enum is declared as “Languages” and the values are declared in the enum.
- In the class created as “ValueOfExample1”, the elements are entered using the ValueOf() method in Java.
- The output value for each element is printed using the println() method along with the toString() method that returns the enum constant name.
Output
The output below shows that the values are declared according to the specified enum.

Example 2: Using the ValueOf() Method to Fetch the Required Value
The code below shows that the ValueOf() method returns the specified enum with the specified value.
import java.lang.*;
// enum showing Students with Marks
enum Student {
John(80), Smith(90),Lilly(85), Adam(87);
//Declare the objects
int marks;
Student(int s) {
marks = s;
}
int showMarks() {
return marks;
}
}
//Declare the class
class ValueOfExample2 {
public static void main(String args[]) {
System.out.println("MARKS LIST:");
//Use the ValueOf() method to fetch the value
for(Student st : Student.values()) {
System.out.println(st + " obtained " + st.showMarks() + " marks");
}
Student mar;
mar = Student.valueOf("Smith");
System.out.println("The Student with highest marks is : " + mar);
}
}
In the above code block:
- The enum is declared with the names of the students and marks.
- The marks and the showMarks are declared as the integer data type.
- In the next step, the class is declared as the “ValueOfExample2”.
- The values() method of enum returns all the values present in the specified enum.
- The println() method of Java prints the student names along with the marks.
- In the last part of the code, the valueOf() method returns the value declared in the enum.
Output
The output below shows that the “MARKS LIST” is declared and the specified value of the enum “Smith” is returned using the valueOf() method.

Bonus Method: The example below shows the implementation of another method to fetch the index of the specified enum termed as “ordinal()” method. This method is implemented with the valueOf() method of enum in Java.
Example 3: Using ordinal() to Fetch the Index
The ordinal() method of the enum in Java is implemented along with the valueOf() method to fetch the index of the specified enum in Java. The code below implements the ordinal() method of enum.
//Declare the class
class ValueOfExample3 {
public enum Student {John, Smith, Lilly, Adam;
}
public static void main(String args[]) {
System.out.println("STUDENT LIST:");
//Use the ValueOf() method to fetch the value
for(Student st : Student.values()) {
System.out.println(st);
}
System.out.println("The Index of Student 'Lilly' is " +Student.valueOf("Lilly").ordinal());
}
}
In the above code block:
- The class is declared as “ValueOfExample3” along with the enums.
- The values() method returns all the values present in the enum.
- In the last step, the ValueOf() method returns the specified value that is “Lilly” along with the ordinal() method which returns the index of the specified enum.
Output
In the output below, the student list is printed along with the index of the student named “Lilly” using the ordinal() method of enum in Java.

Exceptions While Working With the valueOf() Method in Java
There are two exceptions raised while working with the valueOf() method in Java. These are described below.
- Illegal Argument Exception
- NullPointer Exception
These exceptions are implemented through the code examples.
Exception 1: IllegalArgumentException of valueOf() Method
The code below shows that an exception is raised if the value specified in the valueOf() method is not present in the enums declared.
//Declare the class
class ExceptionExample1 {
public enum Student {John, Smith, Lilly, Adam;
}
public static void main(String args[]) {
System.out.println("STUDENT LIST:");
//Use the ValueOf() method to fetch the value
for(Student st : Student.values()) {
System.out.println(st);
}
System.out.println(Student.valueOf("Marie"));
}
}
The above code searches for the value of “Marie” in the enum using the valueOf() method.
Output
The Student list is printed as usual but the exception “IllegalArgumentException” occurred while working with the valueOf() method since the student “Marie” is not present in the declared enum.

Exception 2: NullPointerException in valueOf() Method in Java
The code below shows that if a null is declared in the valueOf() method of enum, the method raises an exception.
//Declare the class
class ExceptionExample2 {
public enum Student {John, Smith, Lilly, Adam;
}
public static void main(String args[]) {
System.out.println("STUDENT LIST:");
//Use the ValueOf() method to fetch the value
for(Student st : Student.values()) {
System.out.println(st);
}
System.out.println(Student.valueOf(null));
}
}
In the above code block, the println() method contains “null” declared with the valueOf() method.
Output
The output below shows that the “NullPointerException” is raised when the code is run with a null value.
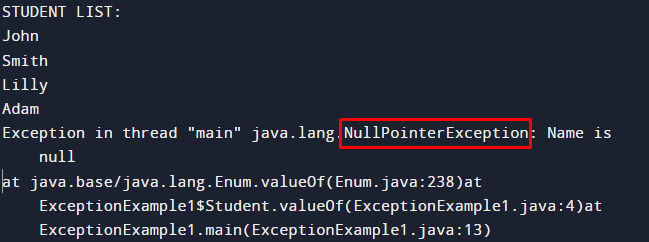
This concludes the working of the valueOf() method of enum in Java.
Conclusion
The valueOf() method of an enum in Java is responsible for fetching a certain value from the specified enum. This method helps to fetch the required enum from the declared enums in Java. In this article, we have discussed different examples along with the exceptions that can arise while implementing the valueOf() method in Java.