Finding the largest element in an array is a crucial task that helps us analyze data effectively. For instance, determining the salary of the highest-paid employee, identifying the student with the highest marks, or calculating the range of values in a dataset. In Java, various methods are available to find the maximum value in an array, such as Arrays.sort(), Collections.max(), recursive approach, etc.
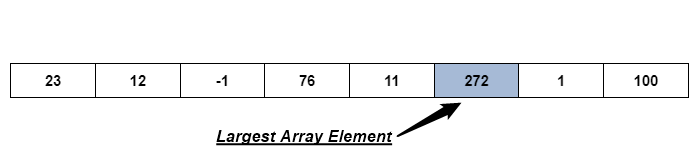
This write-up will present a comprehensive guide on finding and printing the largest array element using different built-in and user-defined methods.
Method 1: Find the Largest Array Element Using For Each Loop
for each loop iterate over each element of the provided array/collection. The loop structure includes an if statement to find the largest array element. For this, initialize an array of your choice, we initialize an integer-type array:
int[] inputArray = {110, 2, 4, 9, 21, 0, -111, 5, 121, 172, 18};
Now create a variable and initialize it with the first element of the array. This means currently we are assuming the first array element is the greatest among all:
int maxNum = inputArray[0];
Execute the for-each loop to iterate every single element of the “inputArray”. Within the loop, check if the current element of the inputArray is greater than the maxNum. If so, update the value of maxNum with the current array element:
for (int currentNum : inputArray) {
if (maxNum < currentNum)
maxNum = currentNum;
}
Finally, display the largest array element on the console using the following line of code:
System.out.println("The Largest Array Element is " + maxNum);
Output

Method 2: Find the Largest Array Element Using Arrays.sort()
The sort() is a built-in method of Java’s Arrays class. It sorts an array of primitive data types or objects in natural/ascending order. Use the sort() method to sort the input array and then print the last element of the sorted array to find the largest array element.
First, import the Arrays class in your program to access any of its methods like “sort()”:
import java.util.Arrays;
Initialize an input array upon which you want to use the sort() method:
int[] inputArray = {110, 2, 4, 219, 225, 0, -111, 521, 121, 172, 281};
Now apply the sort() method on the inputArray to sort it in default ascending order:
Arrays.sort(inputArray);
Finally, print the last element of the sorted array to find the largest element:
System.out.println("The Largest Array Element is " + inputArray[inputArray.length-1]);
Output

Method 3: Find the Largest Array Element Using Java 8 Stream
Java 8 introduced the Stream API which provides an effective way of processing collections of objects. Some of the built-in methods of the stream API can be used to find the largest array element.
To use the Java streams method, first, import the Arrays class from the java.util package:
import java.util.Arrays;
The Java 8 streams deal with the integer data so Initialize an integer-type array:
int[] inputArray = {110, 2, 4, 9, 21, 0, -111, 5, 121, 172, 18};
Now apply the stream methods to get the maximum element in the array and print it on the console as output:
int maxNum = Arrays.stream(arr).max().getAsInt();
System.out.println("The Largest Array Element is " + maxNum);
Output

Method 4: Find the Largest Array Element Using Collections.max()
The max() method of the “Collections” class retrieves the maximum element from the provided collection. To find the largest array element via the “max()” method of the Collections class, first, import the ArrayList and Collections classes from the java.util package:
import java.util.ArrayList;
import java.util.Collections;
Initialize the array whose largest element you want to find:
int[] inputArray = {110, 2, 4, 219, 221, 0, -111, 5, 121, 172, 181};
Now create an ArrayList in which the element of the inputArray will be stored:
ArrayList<Integer> arrlist = new ArrayList<>();
Use a loop structure to iterate over the “inputArray”. Within the loop, use the add() method to add each element of the inputArray to arrList:
for (int i = 0; i < inputArray.length; i++) {
arrList.add(inputArray[i]);
}
Find the largest number in the arrList using the max() method and print it on the console using the println() method:
int maxNum = Collections.max(arrList);
System.out.println("The Largest Array Element is " + maxNum);
Output

Method 5: Find the Largest Array Element Using User-Defined Function
You can create a function and customize a logic to find the largest array element. For instance, we will create a user-defined function and use the iterative approach to find the largest array element. For this purpose, first, initialize an integer-type static array at the class level:
static int[] inputArray = {11, 211, 114, 29, 25, 281, 121};
Create a user-defined static method to find the largest array element. Within the findLargest() method, create an integer-type variable to store the first element of the array (assuming it is the largest array element). Use the for-loop to traverse the “inputArray” up to its length. Within the loop, check if the current array element is greater than maxNum. If yes, update the maxNum with the current element. Finally, return the maxNum:
static int findLargest() {
int maxNum = inputArray[0];
for (int i = 1; i < inputArray.length; i++)
if (inputArray[i] > maxNum)
maxNum = inputArray[i];
return maxNum;
}
Invoke the user-defined findLargest() method from the main() method to find and print the largest array element:
System.out.println("The Largest Array Element is " + findLargest());
Output

Method 6: Find the Largest Array Element Using Recursion
In Java, recursion is a technique where a method invokes itself to solve a specific problem. This process keeps on going until a specified base or termination condition is met. The base/termination criteria must be provided to ensure that the function eventually leads to a solution and to avoid infinite recursion.
As an example, create a recursive function that accepts an input array and array size as parameters. The function returns inputArray[0] if the array has only a single element. In case the input array has more than one element, the Math.max() method will be invoked on each element of the input array recursively. This procedure proceeds until the base condition is met, i.e., “arrSize == 1”.
static int findLargest(int inputArray[], int arrSize){
if(arrSize==1)
return inputArray[0];
return Math.max(inputArray[arrSize-1], findLargest(inputArray, arrSize-1));
}
In the main() method, initialize an array and find its size using the length property:
int[] inputArray = {11, 211, 114, 29, 25, 281, 121};
int arrSize = inputArray.length;
Now invoke the user-defined findLargest() function and pass the inputArray and array size as its arguments:
System.out.println("The Largest Array Element: " + findLargest(inputArray, arrSize));
Output

That’s all about finding and printing the largest array element.
Final Thoughts
In this Java guide, we have explored six different approaches including built-in methods as well as user-defined methods/logics. Initially, we explained how the for each loop is used with the if statement to easily find the largest array element. After this, we demonstrated how fast and effective the Java 8 Stream API, Arrays.sort(), and Collections.max() methods can find the largest element in an array. Finally, we headed toward custom logic, where we learned how to find the largest element from the given array using an iterative or recursive approach.