A Set is an in-built interface of Java’s “util” package. It represents the collection of unique elements (no duplicates). Java allows us to perform several operations on the sets such as Union, Complement, and Intersection.
The “intersection of two sets” is denoted by the “∩” symbol and is defined as a set that contains common elements of both sets. For example, if Set_1 = {12, 5, 14, 7} and Set_2 = {5, 15, 7, 13}, then Set_1 ∩ Set_2 = {5, 7}. The following Venn diagram shows the intersection of set_1 and set_2:

Let’s learn how to get the intersection of two sets in Java using different methods.
How to Get/Calculate Set Intersection in Java?
To get/calculate the intersection of two sets in Java, different methods like “brute force”, “retainAll()”, and “Sets.intersection()” are used. We will discuss all these methods one by one using practical examples.
How to Get/Calculate Intersection of Two Sets in Java Using Nested For Loop (Brute Force)?
Use the nested loops to iterate over each set, and within the inner loop, use the if statement to compare the set elements. If an element is common in both sets, print it on the console.
Example: Calculate Set Intersection Using Nested Loops
Use the following statement at the start of your program to import the required classes and interfaces from the “java.util” package:
import java.util.*;
Create a couple of sets using the “HashSet”:
Set<String> set_1 = new HashSet<>();
Set<String> set_2 = new HashSet<>();
Insert elements to each set using the “add()” method:
//add elements to set_1
set_1.add("Red");
set_1.add("Green");
set_1.add("Yellow");
set_1.add("Orange");
set_1.add("Red");
//add elements to set_2
set_2.add("Red");
set_2.add("Black");
set_2.add("Yellow");
set_2.add("Blue");
set_2.add("Red");
Print elements of both sets on the console:
System.out.println("set_1 is " + set_1);
System.out.println("Set_2 is " + set_2);
Use the nested for-each loop to iterate over both sets. Within the inner loop, use an if statement to find and print the common elements among the given sets:
System.out.println("set_1 ∩ Set_2 = ");
for(String i: set_1) {
for(String j: set_2) {
if (i == j) {
System.out.print(j + " ");
}
}
Output
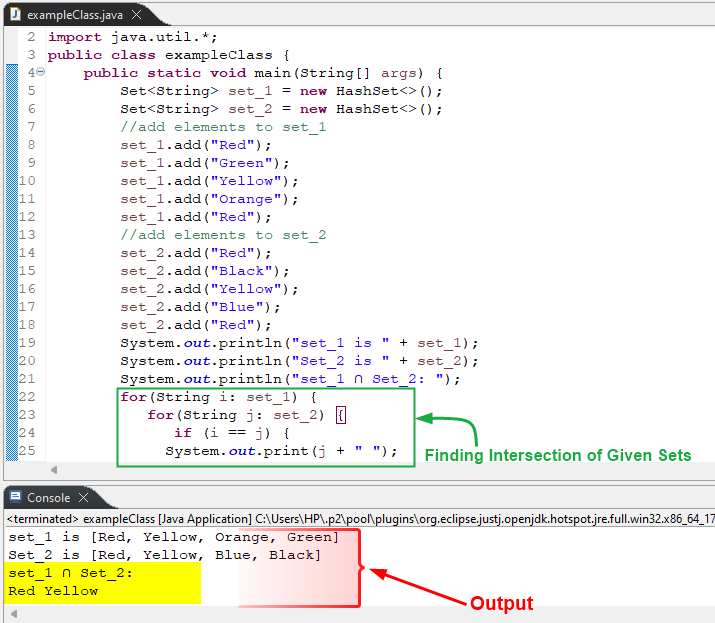
How to Get/Calculate Intersection of Two Sets Using Java retainAll() Method?
The retainAll() is a built-in method of the ArrayList class that accepts a collection as a parameter and compares its element with the selected ArrayList. It retains only those elements that are common in the ArrayList and the provided Collection. You can use this method to get/compute the intersection of given sets.
Example: Find Set Intersection Using retainAll()
Let’s create the sets whose intersection you want to find. In this example, we use the HashSet constructor to create and initialize the desired sets (you can do the same using the add() method as we did in the above example):
List<Integer> list_1 = Arrays.asList(12, 5, 14, 7);
Set<Integer> set_1 = new HashSet<>(list_1);
List<Integer> list_2 = Arrays.asList(5, 15, 7, 13);
Set<Integer> set_2 = new HashSet<>(list_2);
Print the original sets using the println() method:
System.out.println("set_1 is " + set_1);
System.out.println("Set_2 is " + set_2);
Let’s apply the “retainAll()” method on the given sets to find their intersection:
set_1.retainAll(set_2);
Finally, print the intersection of the given sets on the console:
System.out.println("set_1 ∩ Set_2 = "+ set_1);
Output
“5 and 7” are common elements in both the sets, so the intersection of the given sets using the retainAll() method is

How to Get Intersection of Two Sets in Java Using Sets.intersection() Method?
Guava Library offers a “Sets.intersection()” method that returns an unmodifiable set, representing the intersection of two sets. Let’s learn how it works using the following example.
Example: Get Set Intersection Using Sets.intersection()
To use Sets.intersection() method, first, download a jar file for the Guava Library from the official site and add it to your Java project. After this, right-click on the added “jar” file, hover over the “Build Path” option, and click on the “Add to Build Path” option to add this jar file to the build path:

Once the guava library is successfully added to the build path, you can import it into your program using the import statement:
import com.google.common.collect.Sets;
Create the sets, add elements to them, and print them on the console:
Set<String> set_1 = new HashSet<>();
Set<String> set_2 = new HashSet<>();
//add elements to set_1
set_1.add("Red");
set_1.add("Green");
set_1.add("Yellow");
//add elements to set_2
set_2.add("Red");
set_2.add("Black");
//Print set_1 and set_2
System.out.println("set_1 is " + set_1);
System.out.println("Set_2 is " + set_2);
Now use the “Sets.intersection()” method to find the intersection of “set_1” and “set_2”:
System.out.println("set_1 ∩ Set_2: " + Sets.intersection(set_1, set_2));
Output

How to Get Intersection of Multiple Sets in Java?
To get the intersection of multiple sets in Java, create a static-type generic method. This method calculates the intersection of multiple collections and retrieves the result as an unmodifiable set:
public static <T, C extends Collection<T>> C getSetIntersection (C newSet, Collection<T>... collections) {
boolean flag = true;
for (Collection<T> i: collections) {
if (flag ) {
newSet.addAll(i);
flag = false;
} else {
newSet.retainAll(i);
}
}
return newSet;
}
In the above code:
- First, we create a function named “getSetIntersection()”.
- Next, we use the enhanced for each loop to iterate through the given collections.
- In the first iteration, we add the elements of the first collection to the “newSet” and then we set the flag to false. This indicates that the subsequent iterations will perform a set intersection.
- In the else block, we use the retainAll() method to retain only those elements that are common between newSet and the current collection i.
- Finally, return the “newSet” which represents the intersection of all input collections.
public static void main(String[] args) {
List<Integer> list_1 = List.of(5, 12, 14, 72);
List<Integer> list_2 = List.of(72, 75, 5, 100, 110);
List<Integer> list_3 = List.of(1, 3, 5, 12, 15, 72);
List<Integer> list_4 = List.of(1, 5, 72, 100, 120);
Set<Integer> setIntersection = getSetIntersection(new HashSet<>(), list_1, list_2, list_3, list_4);
System.out.println("Intersection of Given Sets: " + setIntersection);
}
In the main() method:
- First, we create four lists of type integer: “list_1”, “list_2”, “list_3”, and “list_4”.
- After this, we call the getSetIntersection() method to compute the intersection of given sets and store the result in a HashSet.
- Finally, we print the intersection of multiple sets on the console using the “println()” method.
Output

That’s all about getting the set intersection in Java.
Conclusion
A set that contains common elements of the two given sets is known as the “intersection of two sets”. To calculate the intersection of two sets in Java, you can use different methods such as “brute force”, “retainAll()”, and “Sets.intersection()”. Among all these methods, the “retainAll()” is the most convenient one. It retains only those elements that are common in both sets. This Java blog has explained how to get the intersection of two sets using all these methods.