In Java, the isNumeric() is a static method of the StringUtils class that checks if the input string has only Unicode digits. It retrieves “true” in the case of Unicode digits only. However, if the given string contains a Unicode symbol like “null”, “decimal point”, or a “leading sign”, then it retrieves “false”.
This guide explains the working of isNumeric() method in Java. Also, it will discuss some suitable alternatives to the stated method.
How to Use the isNumeric() Method in Java?
To use the isNumeric() method in Java, create a Maven project, and add the Apache Commons Lang dependencies in its “pom.xml” file. Doing this will allow you to import the String Utils class in your Java code. Once the String Utils class is successfully imported, follow the following syntax to use the isNumeric() method in your Java code:
public static boolean isNumeric(final CharSequence charSeq);
The isNumeric() accepts only a single parameter “charSeq”, which represents a string to check.
Prerequisite Step
Apache Commons is an external library, therefore, to use it, first, it must be added to the “pom.xml” file of a Maven project, as follows:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.14.0</version>
</dependency>
pom.xml File:

Now import the “StringUtils” class from the Apache Commons library:
import org.apache.commons.lang3.StringUtils;
Example 1: Using isNumeric() With Unicode Digits
In the main() method, initialize a string that contains only Unicode digits and apply the isNumeric() on it:
String inputString = "5121472";
System.out.print(inputString + "Contains only Unicode digits: " + StringUtils.isNumeric(inputString));
Output retrieves true because the inputString contains only Unicode Digits:

Example 2: Using isNumeric() With Whitespaces
Let’s apply the isNumeric() method on an empty string, null, and a string that contains Unicode digits and whitespaces:
String inputString1 = " ";
String inputString2 = "512 1472";
String inputString3 = null;
System.out.println("InputString1 Contains only Unicode digits: " + StringUtils.isNumeric(inputString1));
System.out.println("InputString2 Contains only Unicode digits: " + StringUtils.isNumeric(inputString2));
System.out.println("InputString3 Contains only Unicode digits: " + StringUtils.isNumeric(inputString3 );
The isNumeric() method retrieves “false” for all three input strings. The inputString2 has Unicode digits but contains whitespace between them, so the isNumeric() method retrieves false:

Example 3: Using isNumeric() With Unicode Digits and Symbols
Now initialize a string with Unicode letters, digits, and symbols, and see how the isNumeric() method deals with that string:
String inputString = "5 12-Java-14Beat72";
System.out.println("InputString Contains only Unicode digits: " + StringUtils.isNumeric(inputString));
The presence of the characters and symbols in the inputString results in a boolean false:

Example 4: Using isNumeric() With a Leading Sign or Decimal Point
Let’s implement the isNumeric() method on a string having a leading sign or decimal point, as a result, you will get “false” in the output:
String inputString1 = "+5121472";
String inputString2 = "-5121472";
String inputString3 = "512.1472";
System.out.println("InputString1 Contains only Unicode digits: " + StringUtils.isNumeric(inputString1));
System.out.println("InputString2 Contains only Unicode digits: " + StringUtils.isNumeric(inputString2));
System.out.println("InputString3 Contains only Unicode digits: " + StringUtils.isNumeric(inputString3));
As expected, the isNumeric() method retrieves a boolean false when it encounters a leading sign or a decimal point:
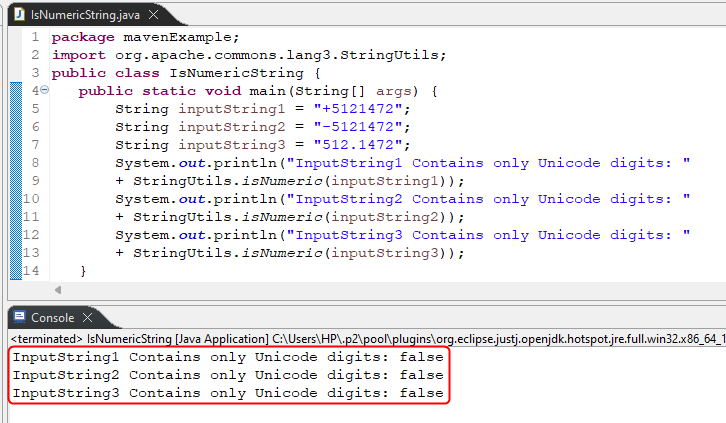
This is how the isNumeric() method works in Java.
Useful Alternatives to Check if a String is Numeric in Java
In Java, there are several alternatives available for the isNumeric() method. Among these alternatives, some are much more flexible than the isNumeric() method. The commonly used methods are illustrated in the following table along with their basic syntax and description:
Method | Syntax | Description |
---|---|---|
isNumericSpace() | StringUtils.isNumericSpace(CharSequence charSeq); | Works similarly to the isNumeric() method, the only difference is it retrieves true for empty strings and strings having integers or whitespaces. |
parseInt() | Integer.parseInt(inputString); | Parses the given numeric string and retrieves an integer. |
parseDouble() | Double.parseDouble(inputString); | Parses the input string and returns a double value. |
parseFloat() | Float.parseFloat(inputString); | It accepts a numeric string and parses it to float. |
parseLong() | Long.parseLong(inputString); | It parses the numeric string into a long value. |
BigInteger() | new BigInteger(inputString); | This constructor converts the given string into a BigInteger. |
isCreatable() | NumberUtils.isCreatable(inputString); | It checks if the input string is numeric. Returns true if numeric, and false otherwise. |
Regular Expressions | string.matches(regexPattern); | Use the regex pattern “-?\d+(\.\d+)?” to check if a string is numeric. |
To learn how these methods work, visit our dedicated guide on how to check if a string is numeric in Java.
Conclusion
In Java, the StringUtils.isNumeric() accepts a string as a parameter and checks if the input string contains all Unicode digits. If yes, it retrieves true, otherwise, false. We considered different use cases for the isNumeric() method and concluded that it retrieves true if and only if the given string contains Unicode digits. This means isNumeric() is less flexible as it retrieves false for the “null” strings, empty strings, “decimal points”, and “leading signs”.
Considering all these facts and figures, it is recommended to use the isNumeric() method only if you are checking for positive integers in a string. However, if you want to check if a string is numeric with a bit more flexibility, use any other alternative, like regex, parsing, etc.