In Java, the total number of rows determines the length of a 2D array. For this, the length property is used in conjunction with the array name i.e., arrayname[row].length. In a fixed-size 2d array, each row consists of an equal number of columns. However, finding the length of uneven or jagged 2D arrays can be challenging when the elements are initialized individually.
Read more: Java 2D array
Quick Outline
This article discusses the following:
- Find the Length of a Fixed 2D Array
- Find the Length of an Uneven 2D Array
- Find the Length of a Non-Primitive 2D Array
The length property determines the total rows of fixed or jagged multidimensional arrays and can also be used to get the length of each dimension individually. Oftentimes, users confuse the functionalities of length, length(), and size() in Java as they seem to be closely related. However, the three differ greatly as shown by the table given below:
length | length() | Size() |
---|---|---|
The length is a property used with the array. | The length() is a method used with Strings. | The size() is a method of the Collections class which is used with ArrayLists. |
It returns the number of elements in one-dimensional arrays or returns the length of rows or columns in multidimensional arrays. | The String class length() function returns the character count of a string. | The size() method counts the total elements within an ArrayList and returns the number. |
Now, let’s learn to find the 2D array length in Java.
How to Get the Length of a Fixed-Size 2D Array in Java?
The 2D array can be perceived as a table that contains rows and columns. In fixed two-dimensional arrays, the number of columns per row remains the same. For instance, in a 2D array of 3 x 6, there are 3 rows and 6 columns per row:
In this code, a 2D integer array is created with the given values. The “numArray.length” returns the total rows and the “numArray[0].length” returns the total columns of the 0th row:
int [ ] [ ] numArray= {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12} };
//length of 2D array is total number of rows
System.out.println("Rows are : " + numArray.length + " and Columns are " + numArray[0].length);
To get the total number of elements (size) in the fixed-size 2D array, multiply the total row count by the total columns of the 0th row. As it is a fixed-sized array, the number of columns per row will remain the same. The code is given as follows:
//Total number of Elements
System.out.println("Total elements are : " + numArray.length * numArray[0].length);
Complete Code & Output
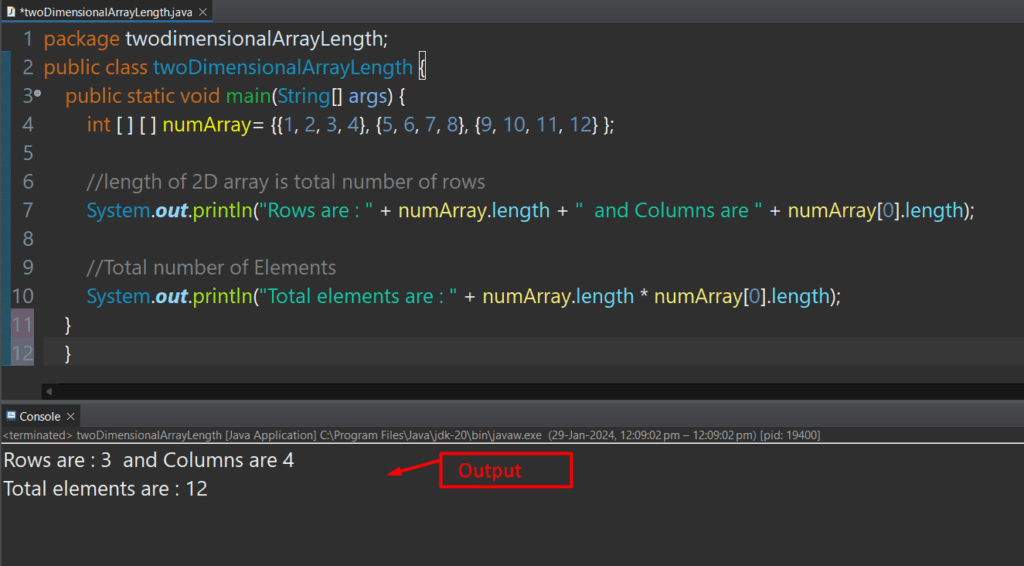
How to Get the Length of an Uneven 2D Array?
The “uneven” or a “jagged” 2D array is a multi-dimensional array that contains different numbers of columns per row.

The “numArray” is an uneven 2D array and the “row” is an integer variable. The for loop traverses array’s elements and terminates when “i” is greater than the numArray’s length. The length of each row is returned by numArray[i].length and is displayed. The “row” variable is incremented by 1 to move to next row of the 2D jagged array:
int [] [] numArray= {{1, 2, 3,}, {4, 5}, {6, 7, 8, 9}, {11}}; //2D uneven array
int row=1;
for(int i=0; i<numArray.length;i++)
{
System.out.println(" Row " + row + " has : " + numArray[i].length + " columns");
row=row+1;
}
Complete Code & Output
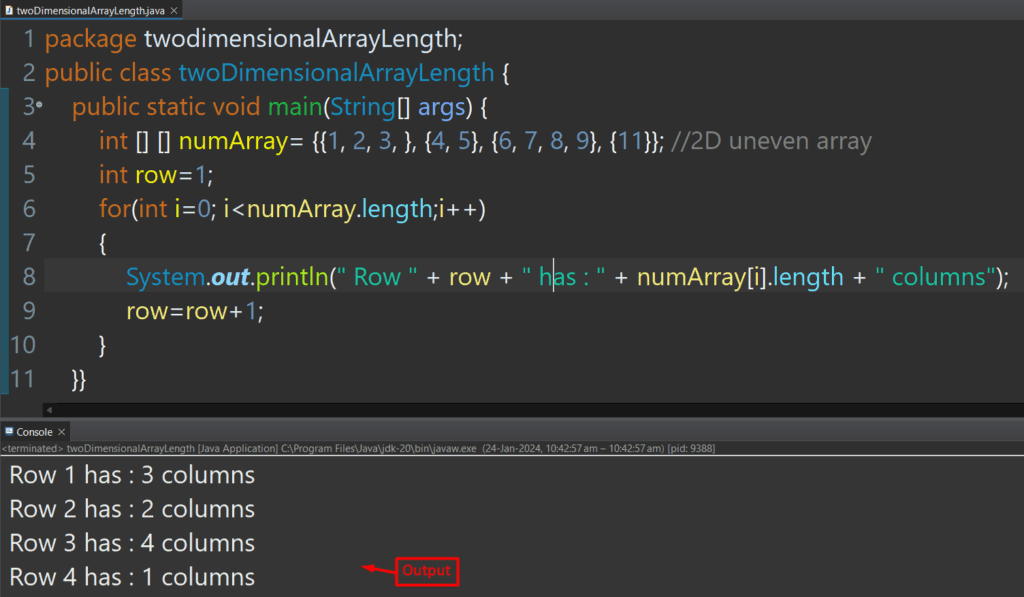
Bonus Tip: Find the Size of an Uneven 2D Array
To calculate the size of a jagged 2D array, a while loop is used that iterates over the elements of the array. The integer variable “len” adds and stores the number of columns of the current row (numArray[i].length). The loop variable “i” is incremented to move to the next row in the 2D array. Finally, the output is printed:
int [] [] numArray= {{1, 2, 3, }, {4, 5}, {6, 7, 8, 9}, {11}}; //2D uneven array
int i=0, len=0;
while(i<numArray.length)
{
len=len+numArray[i].length;
i++;
}
System.out.println("Total Elements are: " + len);
Complete Code & Output

How to Get the Length of a Non-Primitive 2D Array?
In Java, the non-primitive data types can store multiple values and are derived from primitive data types such as an array of integers or a string of characters, etc. The non-primitive data types are instances of the classes.
To determine the length of a non-primitive 2D array, create an object array of fixed rows and columns. The “objArray.length” returns the row count of the array. As it is a fixed-size array, the number of columns per row remains the same. The columns per row are determined by the “objArray[0].length” as shown in the code below:
Object[ ] [ ] objArray= new Object[3][4];
System.out.println("Rows are : " + objArray.length + " and Columns are " + objArray[0].length);
Note: The size of the fixed-size non-primitive 2D array can be determined in a similar method as shown in the “How to Get the Length of a Fixed Size 2D array” of this article.
Similarly, another Object array “objArray2” is declared that has a different number of columns per row. The “row” variable is initialized with the value “1” to indicate the current row. The for loop iterates through the array’s elements and terminates when “i” is greater than the length of the array. The print statement displays the length of each row and the “row” variable is incremented in each iteration:
//Length of Jagged 2D array
Object [ ] [ ] objArray2= { {1, 2 }, {3, 4, 5}, { 6 } };
int row=1;
System.out.println("Length of a Jagged/Uneven 2D array");
for (int i=0; i<objArray2.length;i++ )
{
System.out.println("Row " + row + " has : " + objArray2[i].length + " columns");
row = row+1;
}
Note: The size of the uneven non-primitive 2D array can be determined in a similar method as shown in the “How to Get the Length of an Uneven 2D array” of this guide.
Complete Code & Output

That is all from this guide.
Conclusion
In 2D arrays, the elements are stored in contiguous memory locations in the form of rows and columns. Determining the total length of the 2D array can be challenging as the number of columns per row can vary. To find the 2D array length, use “arrayname[i].length” which will return the length of each column per row as shown in this guide. This article is a practical guide for finding the length of the 2D array in Java.