In Java, containers i.e., Array, ArrayList, HashMap play a vital role in containing the data of various data types. A “2D array”, however, comes into effect when there arises a need of sorting the data, especially in the case of a large set of data. This feature arranges this data in the rows and columns that can also be accessed conveniently.
This article will demonstrate the concept of the Java “2D array”.
What is a Java 2D Array?
A two dimensional array corresponds to the simplest form of a multidimensional array that is an array of one dimensional array. It is such that it comprises two indices, one corresponds to rows and the other to a particular column in that row.
Syntax (2D Array Declaration)
Datatype[][] array;
In this syntax, “Datatype” refers to the array’s data type and “array” is the array’s name.
Syntax (2D Array Creation)
Array = new Dt[r][c];
In the above syntax:
- “Dt” indicates the array’s data type.
- “r” is the array’s rows.
- “c” points to the array’s columns.
Syntax with Example
int[][] x = new int[2][3];
Here, a 2D array named “x” is created that can comprise a maximum of “2 * 3 -> 6” elements.
Syntax (Declaring a 2D array with one dimension)
int[][] a = new int[2][];
This syntax declares a 2D integer array comprising two rows and an undefined number of columns.
Example 1: Initializing the 2D Array and Iterating Along the Array
In this example, a “2D” array will be initialized and iterated:
public class Array2D {
public static void main(String[] args) {
int[][] array2D = {{1, 2},
{3, 4, 5},
{6, 7, 8, 9}};
for (int i = 0; i<array2D.length;i++)
for(int j = 0;j<array2D[i].length;j++)
System.out.println(array2D[i][j]);
}}
In this snippet of code:
- Initialize a 2D integer array without size allocation. It is such that it can comprise any number of rows and columns.
- This array contains the provided integers allocated in a custom manner as rows and columns.
- Now, the first “for” loop iterates along the array rows and the second nested “for” loop iterates through the elements in those rows.
Output

As seen, all the array elements are iterated and returned.
Example 2: Initializing a Defined Array by Adding the Elements via Indexing
In this demonstration, a “2D” array will be initialized with a predefined set of rows and columns and then accessed via indexing:
public class Array2D {
public static void main(String[] args) {
int[][] array2D = new int[2][3];
array2D[0][0] = 1;
array2D[0][1] = 2;
array2D[0][2] = 3;
array2D[1][0] = 4;
array2D[1][1] = 5;
array2D[1][2] = 6;
for (int i = 0; i<array2D.length;i++)
for(int j = 0;j<array2D[i].length;j++)
System.out.println(array2D[i][j]);
}}
According to these lines of code:
- Create a 2D integer array having 2 rows and 3 columns.
- Now, add the stated integers in the array via indexing one by one.
- It is such that the first index refers to the rows and the second index points to the columns in the array.
- Lastly, likewise, apply the nested “for” loop to invoke all the 2D array elements and return them.
Output
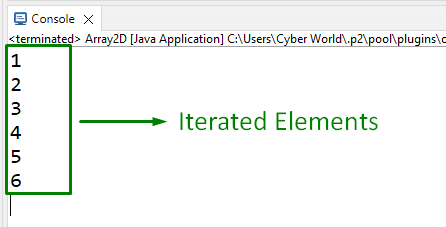
Another approach to access the array elements individually can be via indexing demonstrated as follows:

This outcome implies that the indexed element is retrieved accordingly.
Conclusion
A two dimensional array is an array of one dimensional array that comprises two indices, one corresponds to rows and the other to a particular column in that row. This array can be initialized in a custom manner or based on the predefined set of rows and columns.