Java provides great functionality for the developers to format the values according to default or the specified locale. It is such that various types of currency formats can be retrieved against the input values as per the requirements.
This tutorial will demonstrate the methodologies for formatting decimals in currency format in Java.
How to Format Decimals in a Currency Format in Java?
The decimals can be formatted in a currency format via the “getCurrencyInstance()” method of the “NumberFormat” class. This method returns the currency format for the default or target locale.
Syntax
NumberFormat getCurrencyInstance()
NumberFormat getCurrencyInstance(Locale loc)
In the latter syntax, “loc” corresponds to the locale based on which the formatting is carried out.
Returned Value
It gives a “NumberFormat” instance for currency formatting.
Example 1: Formatting a Decimal in Currency Format
This example formats the specified decimal based on the default locale of the system:
import java.text.*;
public class FormatDecimals {
public static void main(String[] args) {
NumberFormat x = NumberFormat.getCurrencyInstance();
double dec = 237.56;
String formatDec = x.format(dec);
System.out.println("Formatted Decimal in currency format -> "+formatDec);
}}
In this code:
- First, import the “java.text.*” package that is basically used for formatting purposes.
- After that, apply the “getCurrencyInstance()” method of the “NumberFormat” class to fetch the currency instance.
- In the next step, initialize the decimal to be formatted.
- Lastly, use the “format()” method to format the specified decimal with respect to the fetched currency instance.
Output

Example 2: Formatting a Decimal in Currency Format Based on the Specified Locale
In this particular demonstration, the decimal will be formatted with respect to the specified locale:
import java.text.*;
import java.util.*;
public class FormatDecimals {
public static void main(String[] args) {
NumberFormat x = NumberFormat.getCurrencyInstance(Locale.ITALY);
double dec = 237.56;
String formatDec = x.format(dec);
System.out.println("Formatted Decimal in Italy's currency format -> "+formatDec);
}}
In this block of code:
- Include an additional “java.util.*” package to access all the classes in the “java.util” package.
- After that, apply the “getCurrencyInstance()” method that includes the target locale as its argument based on which the formatting of the decimal needs to be carried out.
- Finally, define the decimal value and format it based on Italy’s currency format via the “format()” method.
Output
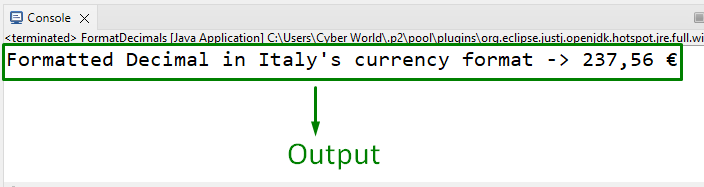
From this output, it can be analyzed that the currency format is changed accordingly.
Conclusion
The decimals can be formatted in currency format via the “getCurrencyInstance()” method of the “NumberFormat” class based on the default or the specified locale. This write-up discussed the methodologies to format decimals in a currency format in Java.