In Java, the operators play a key role in evaluating the initialized or user input values and computing the mathematical expressions as well. The “!=” is one such comparison operator that compares the provided values and returns the corresponding outcome as a Boolean or can be used in loops as well.
What Does != Mean in Java?
The “!=” corresponds to the not equal comparison operator that returns a Boolean outcome “true” if the left operand does not equal the right operand and “false” otherwise.
Example 1: Applying Java “!=” Operator
This example applies the “!=” operator to evaluate the initialized integers and return the corresponding Boolean outcome:
public class Notequaloper {
public static void main(String[] args) {
int x = 3;
int y = 5;
boolean out = x!=y;
System.out.println("Are the Values Equal ? " +out);
}}
In this code:
- Initialize the stated integers that need to be checked for inequality.
- Now, apply the “!=” operator to check if both the defined values are not equal and store the result in the “Boolean” type variable.
- Lastly, return the corresponding Boolean outcome.
Output

This outcome indicates that the Boolean value “true” is returned against the applied “!=” operator since the values are unequal.
Example 2: Applying Java “!=” Operator With “if/else” Statement
In this specific example, the discussed operator can be implemented with an “if/else” statement to invoke the corresponding condition:
public class Notequaloper {
public static void main(String[] args) {
int x = 3;
int y = 5;
if (x!=y)
System.out.println("The values " +x+ " and " +y+ " are not equal!");
else
System.out.println("The values " +x+ " and " +y+ " are equal!");
}}
According to these code lines:
- Likewise, initialize the given integers.
- After that, apply the “!=” operator in the “if” statement to verify if both values are unequal.
- If so, the “if” condition executes. Else, the “else” condition will come into effect.
Output

Example 3: Applying Java != Operator Upon the User Input Values
In this particular demonstration, the “!=” operator will be applied to evaluate the user input values for inequality:
import java.util.Scanner;
public class Notequaloper {
public static void main(String[] args) {
Scanner x = new Scanner(System.in);
System.out.println("Input the first value: ");
int a = x.nextInt();
System.out.println("Input the second value: ");
int b = x.nextInt();
if (a!=b)
System.out.println("The values " +a+ " and " +b+ " are not equal!");
else
System.out.println("The values " +a+ " and " +b+ " are equal!");
x.close();
}}
According to this block of code:
- Import the “java.util.Scanner” package to enable user input.
- Create a Scanner object to allow user input.
- Now, input two values from the user via the “nextInt()” method.
- After that, apply the not equal “!=” operator to check if both the user input values are not equal and invoke the corresponding “if/else” condition.
Output
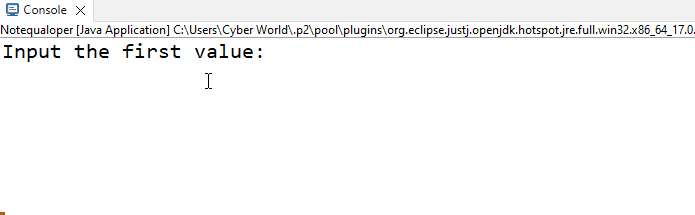
In this outcome, both scenarios (values being equal and not) are demonstrated accordingly.
Conclusion
The “!=” corresponds to the “not equal” operator that returns a Boolean outcome “true” if the left operand does not equal the right operand and “false” otherwise. This tutorial demonstrated the concept and working of the Java “!=” operator.