“Unicode Characters” in programming refers to universal character encoding standards. These characters correspond to the approaches using which the characters can be represented in various documents such as web pages, text files, etc. The three different types of character encodings include “UTF-8”, “UTF-16”, and “UTF-32”.
This article will discuss the approaches to storing “Unicode Characters” in Java.
How to Store Unicode Characters in Java?
The Unicode characters in Java can be stored via the below-given approaches:
- Allocating Unicode to char Data Types.
- Allocating Unicode Values to char Data Types.
- Allocating ASCII Values to char Data Types.
Approach 1: Storing Unicode Characters by Allocating/Assigning Unicode to the char Data Types
In this approach, the Unicode characters can be stored by assigning them to the “char” data type:
public class Unicode {
public static void main(String[] args){
char char1 = 'B';
System.out.println(char1);
char char2 = 'b';
System.out.println(char2);
char char3 = '2';
System.out.println(char3);
char char4 = '%';
System.out.println(char4);
char char5 = '$';
System.out.println(char5);
}}
In the above code lines, contain various sorts of Unicode characters in a “char” type variable and display them accordingly.
Output

As seen, the defined characters are displayed accordingly.
Approach 2: Storing Unicode Characters by Allocating/Assigning Unicode Values to the char Data Types
This approach utilizes the Unicode values instead to store the Unicode characters:
public class Unicode {
public static void main(String[] args){
char char1 = '\u0042';
System.out.println(char1);
char char2 = '\u0062';
System.out.println(char2);
char char3 = '\u0032';
System.out.println(char3);
char char4 = '\u0025';
System.out.println(char4);
char char5 = '\u0024';
System.out.println(char5);
}}
In this block of code, specify the stated Unicode values in a “char” type variable and return them.
Output

Approach 3: Storing Unicode Characters by Allocating/Assigning ASCII Values to char Data Types
This approach specifies the ASCII values equivalent to the corresponding Unicode characters:
public class Unicode {
public static void main(String[] args){
char char1 = 66;
System.out.println(char1);
char char2 = 98;
System.out.println(char2);
char char3 = 50;
System.out.println(char3);
char char4 = 37;
System.out.println(char4);
char char5 = 36;
System.out.println(char5);
}}
In this code, initialize the “char” type variable with the stated ASCII values representing the corresponding Unicode characters and display the outcome.
Output
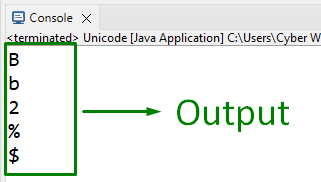
This outcome implies that the characters are generated accordingly.
Conclusion
The “Unicode Characters” in Java can be stored by allocating Unicode, Unicode values, or ASCII values to the “char” data types. This write-up demonstrated different approaches to storing “Unicode Characters” in Java.