In Java, the developers often require to analyze the data types of the appended code features especially while dealing with a complex set of data. This identification assists in applying the code functionalities accordingly. In such a case, Java provides some alternative approaches to identify the type of the values as well as analyzing the relationship between the class objects.
How to Use typeof in Java?
There is no “typeOf” operator in Java. However, some alternative approaches can be considered to return the type of the values. These include the following:
- “getClass()” Method.
- “instanceof” Operator.
- “isInstance()” Method.
Approach 1: Return the Type Using the “getClass()” Method
The “getClass()” method retrieves the class name of the object. This method can be applied with the values of various data types to return their corresponding classes:
public class Typeof {
public static void main(String args[]) {
Integer in = 3;
System.out.println("Integer -> "+in);
System.out.println("Type -> "+in.getClass());
String st = "Linuxhint";
System.out.println("String -> "+st);
System.out.println("Type -> "+st.getClass());
Character ch = 's';
System.out.println("Character -> "+ch);
System.out.println("Type -> "+ch.getClass());
}}
In this code, initialize the integer and return its class via the applied “getClass()” method. After that, repeat the same with both the string and character values as well.
Output

This output confirms that the corresponding classes are retrieved appropriately.
However, the “getSimpleName()” can also be associated with the discussed method to only retrieve the type as a single string, demonstrated as follows:
Approach 2: Return the Type Using the “instanceof” Operator
In Java, the “instanceof” operator analyzes if an object is an instance of the particular type, i.e., class, interface etc. This operator compares the instance with the type and gives “false” upon being utilized with a variable comprising a null value:
public class Typeof{
public static void main(String args[]){
Typeof c=new Typeof();
System.out.println(c instanceof Typeof);
}}
In these code lines, create a class object. After that, utilize the “instanceof” operator to analyze if the object is the instance of the “Typeof” class and return the corresponding Boolean outcome.
Output

This Boolean value implies that the object is an instance of the class.
Approach 3: Return the Type Using the “isInstance()” Method
The “isInstance()” method verifies if the particular object is compatible with the object represented via class. This code example uses this method to check if various class objects correspond to a particular class object:
public class Typeof{
public static void main(String args[]){
Class x = Integer.class;
Integer in = new Integer(22);
Double dbl = new Double(2326565.1);
boolean out = x.isInstance(in);
System.out.println(in + " is Integer? " + out);
out = x.isInstance(dbl);
System.out.println(dbl + " is Integer? " + out);
}}
According to this block of code, perform the following steps:
- Create a class object referring to the “Integer” class.
- Now, create the Integer and Double class objects, respectively comprising the given values.
- Now, apply the “isInstance()” method twice to analyze if these objects are compatible with the referenced class object i.e., Integer and the corresponding Boolean outcome is retrieved in both the cases.
Output
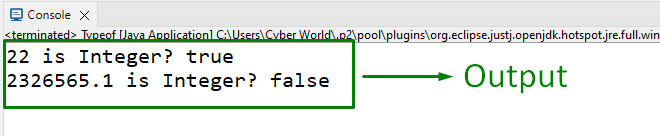
From this output, it can be verified that the “Integer” class object is compatible with the referenced class while it is not the case with the “Double” class object.
Conclusion
The “typeOf” operator in Java does not exist. However, the “getClass()” method, the “instanceof” operator, or the “isInstance()” method can be considered to retrieve the type of the values. Additionally, the “getSimpleName()” method can be utilized to only fetch the type as a single string.