Servlets are small programs that run at server side and creates dynamic web pages. Servlets respond to requests sent by the user. In MVC architecture, servlets act as a controller. The controller processes and responds to user requests. The Servlet Life Cycle contains the following steps:
- Load servlet class.
- Create a servlet instance.
- Call the init method.
- Call the service method.
- Call the destroy method.
Servlet Life Cycle: Class Loading
The first step in the creation of a servlet component is to load the servlet class file into the web container’s JVM (Java Virtual Machine). When the web.xml invokes or configures a first-time servlet with a load-on-startup element, it invokes this step.
Creating Servlet Instance
After loading the servlet class into the web container’s JVM, create an instance of that class. Servlet specification creates only one servlet instance for a single definition in the deployment descriptor.
The init () method
The web container initializes the parameters specified in the deployment descriptor. A servlet first loads into memory, invoking the init () method. The syntax of the init () method looks like this:
[code lang="java"]
public void init () throws Servlet Exception {
//code
}
[/code]
The service () method
After initializing the servlet component, the web container begins sending requests to that component utilizing the service method. The service method processes the request. The web container issues a unique request and response to the service method. The syntax of the service () method:
[code lang="java"]
public void service (Servletrequest request, Servletresponse response)
throws ServletException, IOException {
}
[/code]
When the web container calls the service () method, it invokes the doGet (), doPost (), doPut (), doDelete (), doTrace (), doOptions (), getLastModified () methods.
The doGet () and doPost () are most frequently used with each service request. We must override doGet () and doPost () methods depending on the type of request.
The doGet () method: We use doGet () method to send a specific amount of data. The address bar displays the data. Override doGet () method depending on the type of request. Define as follows:
[code lang="java"]
public void doGet (HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException{
//code
}
[/code]
The doPost () method: Send a large amount of data using the doPost () method. Data is not viewable in the address bar when using this method. The doPost () method sends secure data like passwords. Override the doPost () method depending on the type of request. Define as follows:
[code lang="java"]
public void doPost (HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException{
//code
}
[/code]
doDelete (): is used to delete files, web pages, or documents from the server. It will return HTTP “Bad Request” error if requests are formatted incorrectly. Example:
[code lang="java"]
protected void doDelete (HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException{
//code
}
[/code]
doPut(): Puts files, web pages, or documents in the server for uploading files. If requests are formatted incorrectly then it will return an HTTP “Bad Request” error. Example:
[code lang="java"]
protected void doPut (HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException{
//code
}
[/code]
doTrace (): Logs and debugs. It also tests the requested message. There is no need to override this method. For example:
[code lang="java"]
protected void doTrace(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException{
//code
}
[/code]
doOptions (): Handles the OPTIONS request. There is no need to override this method. It determines which HTTP method is supported by the server and returns the correct header. If requests are formatted incorrectly then it will return an HTTP “Bad Request” error.
For example:
[code lang="java"]
protected void doOptions(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException{
//code
}
[/code]
getLastModified (): Returns what time the request was last modified. It should override a GET request to return the modification time of an object.
For example:
[code lang="java"]
protected long getLastModified (HttpServletRequest request)
throws ServletException, IOException{
//code
} [/code]
The destroy () method
When a web application shuts down, the web container calls the destroy method. The destroy method cleans up any resources that servlet might have initialized. Example of a destroy () method syntax:
[code lang="java"]
public void destroy(){
//code
}
[/code]
Servlet Life Cycle: Example
Now, we will create a servlet example using Eclipse IDE. To create a servlet application, use the following steps:
Environment used:
JDK
Eclipse IDE
Apache Tomcat Server
Java-api
Open the Eclipse IDE and Go to File Menu ->New->Select Dynamic Web Project.
Enter Project Name. Click Next.
Click on Generate web.xml deployment descriptor and click Finish.
Under the project, click Java Resources ->select src, right mouse click, and select New->Package to type the name as javabeat.net.servlets
Now click on the package javabeat.net.servlets and New ->select Servlet
Type the class name as ServletDemo and click Finish.
Now, add a servlet-api.jar to project with the following steps.
- Right click on Project name, select -> Properties ->select Java Build Path-> Click Add External JARs.
- Select servlet-api.jar from the Apache Tomcat directory.
- Hit Open and click OK.
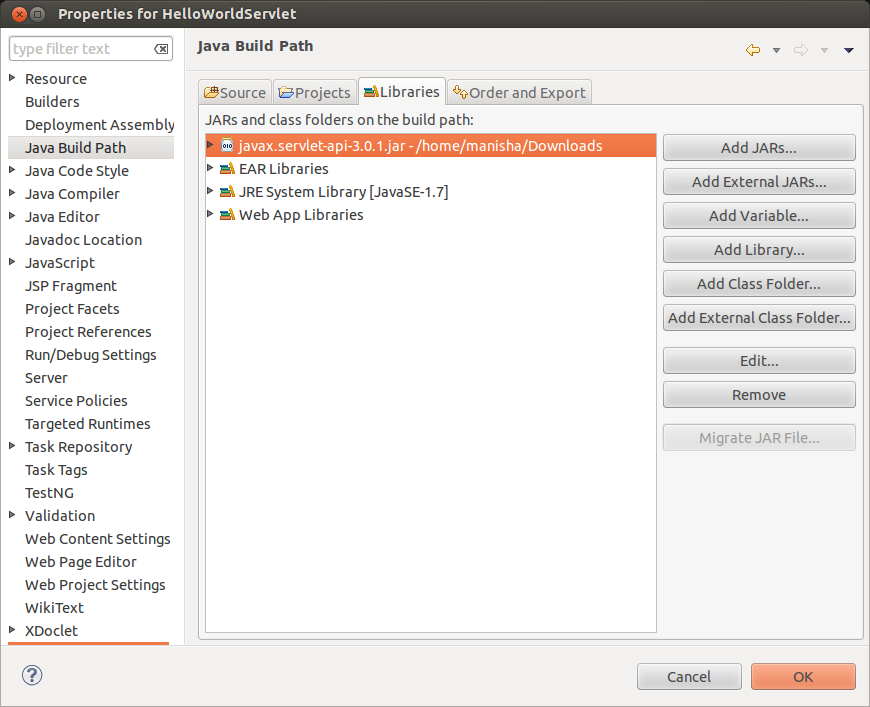
Add the following content to the ServletDemo.java:
[code lang="java"] package javabeat.net.servlets; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Servlet implementation class ServletDemo */ public class ServletDemo extends HttpServlet { /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); PrintWriter out = response.getWriter(); out.println("<html>"); out.println(" <head>"); out.println(" <title>SimpleServlet</title>"); out.println(" </head>"); out.println(" <body>"); out.println(" Hello, World"); out.println(" </body>"); out.println("</html>"); } } [/code]
Edit the web.xml and add the following content:
[code lang="xml"] <?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <servlet> <servlet-name>ServletDemo</servlet-name> <servlet-class>javabeat.net.servlets.ServletDemo</servlet-class> </servlet> <servlet-mapping> <servlet-name>ServletDemo</servlet-name> <url-pattern>/ServletDemo</url-pattern> </servlet-mapping> </web-app> [/code]
Select the class ServletDemo. Right click->Run As and select Run on Server, and you should achieve the output shown below:

- Java EE Tutorials
- Servlets Tutorials
- Servlets Interview Questions
Previous Tutorial: Servlet API || Next Tutorial: Generic Servlet and HTTP Servlet